人脸识别性别年龄python代码
时间: 2023-07-02 14:07:06 浏览: 57
以下是使用Python和OpenCV库实现人脸识别、性别识别和年龄识别的代码:
```
import cv2
import math
import argparse
# 加载人脸识别模型和性别识别模型
face_model = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
gender_model = cv2.dnn.readNetFromCaffe('deploy_gender.prototxt', 'gender_net.caffemodel')
age_model = cv2.dnn.readNetFromCaffe('deploy_age.prototxt', 'age_net.caffemodel')
# 解析命令行参数
parser = argparse.ArgumentParser()
parser.add_argument('--image', help='输入图片路径')
args = parser.parse_args()
# 加载输入图片
img = cv2.imread(args.image)
# 缩放图片
scale_percent = 60
width = int(img.shape[1] * scale_percent / 100)
height = int(img.shape[0] * scale_percent / 100)
dim = (width, height)
img = cv2.resize(img, dim, interpolation = cv2.INTER_AREA)
# 转换为灰度图像
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 检测人脸
faces = face_model.detectMultiScale(gray_img, scaleFactor=1.1, minNeighbors=5)
# 对每个人脸进行性别识别和年龄识别
for (x, y, w, h) in faces:
# 提取人脸图像
face_img = img[y:y+h, x:x+w].copy()
# 进行性别识别
gender_blob = cv2.dnn.blobFromImage(face_img, 1, (227, 227), (78.4263377603, 87.7689143744, 114.895847746), swapRB=False)
gender_model.setInput(gender_blob)
gender_preds = gender_model.forward()
gender = 'Male' if gender_preds[0][0] > gender_preds[0][1] else 'Female'
# 进行年龄识别
age_blob = cv2.dnn.blobFromImage(face_img, 1, (227, 227), (78.4263377603, 87.7689143744, 114.895847746), swapRB=False)
age_model.setInput(age_blob)
age_preds = age_model.forward()
age = int(age_preds[0][0] * 100)
# 在图像上绘制人脸框和性别、年龄信息
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
label = "{} {}".format(gender, age)
cv2.putText(img, label, (x, y), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示输出图像
cv2.imshow('Output', img)
cv2.waitKey(0)
```
说明:
- 代码中使用了OpenCV和argparse两个Python库。
- 人脸识别模型使用了OpenCV自带的哈尔级联分类器。
- 性别识别和年龄识别模型使用了Caffe深度学习框架。
- 代码中使用了命令行参数,可以通过命令行传入输入图片路径。
相关推荐
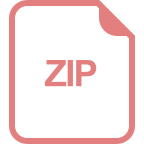
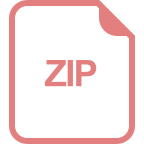
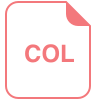
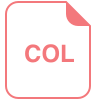
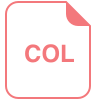
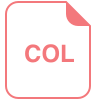
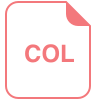









