python 爬取,通过requests爬取豆瓣排行榜的电影名称数据
时间: 2023-10-02 09:11:58 浏览: 131
可以使用requests和BeautifulSoup库来实现这个功能。以下是一个示例代码:
```python
import requests
from bs4 import BeautifulSoup
url = "https://movie.douban.com/chart"
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, "html.parser")
movies = soup.select(".pl2 a")
for movie in movies:
print(movie.text.strip())
```
首先,我们定义一个URL变量,指向豆瓣电影排行榜页面。然后,我们使用requests发送GET请求,获取页面的HTML内容。接下来,我们使用BeautifulSoup解析HTML内容,从中提取出电影名称。最后,我们使用for循环遍历所有电影,并打印出名称。
相关问题
用Python爬取豆瓣网的电影排行榜
您好!以下是用Python爬取豆瓣网的电影排行榜的步骤:
1. 打开豆瓣电影排行榜页面:https://movie.douban.com/chart
2. 分析页面结构,找到电影排行榜所在的HTML标签。可以使用Chrome浏览器的开发者工具来帮助分析。
3. 使用Python的requests库向豆瓣电影排行榜页面发送HTTP请求,并将响应内容解析为BeautifulSoup对象。
4. 使用BeautifulSoup对象的find_all方法找到所有电影的信息所在的HTML标签。
5. 遍历每个电影的信息标签,提取出电影名称、评分等信息,并保存到文件或数据库中。
下面是一段示例代码:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://movie.douban.com/chart'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
movies = soup.find_all('div', class_='pl2')
for movie in movies:
name = movie.find('a').text.strip()
rating = movie.find('span', class_='rating_nums').text.strip()
print(f'{name} {rating}')
```
这个示例代码只是简单地输出每个电影的名称和评分,您可以根据需求进行修改和扩展。希望能帮到您!
python爬取豆瓣评论_python+requests爬取豆瓣歌曲评论
好的,以下是爬取豆瓣歌曲评论的Python代码示例:
首先,我们需要导入需要的库:requests、BeautifulSoup和re。
```python
import requests
from bs4 import BeautifulSoup
import re
```
然后,我们需要定义一个函数来获取评论。这个函数将获取豆瓣歌曲页面上的所有评论,并返回一个评论列表。
```python
def get_comments():
url = 'https://music.douban.com/subject/34926236/comments'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
comments = []
pattern = re.compile('<p class="">(.*?)</p>', re.S)
results = re.findall(pattern, str(soup))
for result in results:
comment = result.strip()
comments.append(comment)
return comments
```
接下来,我们可以调用这个函数,并打印出所有的评论。
```python
comments = get_comments()
for comment in comments:
print(comment)
```
这样就可以爬取豆瓣歌曲评论了。需要注意的是,豆瓣有反爬虫机制,如果请求过于频繁,可能会被封禁IP。因此,在实际应用中,我们需要控制爬取频率,建议使用代理IP。
阅读全文
相关推荐
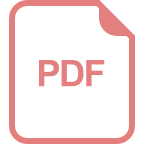
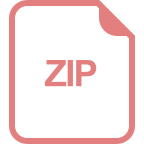


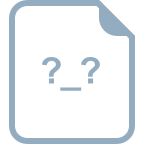
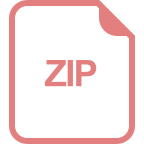
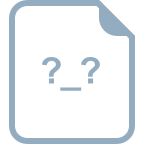
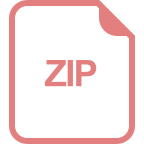
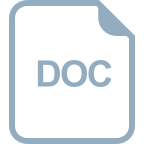






