vector<vector<string>>作为函数参数时应该注意什么,并举例
时间: 2023-12-11 14:02:26 浏览: 36
当将 `vector<vector<string>>` 作为函数参数时,需要注意以下几点:
1. 确定函数参数类型为引用类型,以避免在函数调用过程中进行不必要的拷贝操作。
2. 确定函数参数类型为常量引用类型,以避免在函数内部修改函数参数。
下面是一个例子,假设我们需要实现一个函数来打印一个二维字符串数组:
```
void printStringArray(const vector<vector<string>>& arr) {
for (const auto& row : arr) {
for (const auto& col : row) {
cout << col << " ";
}
cout << endl;
}
}
```
在上面的函数中,我们将 `vector<vector<string>>` 定义为常量引用类型,并使用 `const auto&` 来遍历数组的每个元素。这样可以避免在函数内部修改函数参数,并且可以避免在函数调用过程中进行不必要的拷贝操作。
相关问题
vector<vector<string>>作为函数参数时应该注意什么
当使用vector<vector<string>>作为函数参数时,可以考虑以下几点:
1. 使用引用传递:由于vector是一个动态数组,如果直接使用传值方式,会复制整个数组,造成不必要的开销,因此建议使用引用传递。
2. 确保二维数组的大小:在函数中对二维数组进行操作时,需要确保其大小,否则可能会导致访问越界。
3. 确保内部vector的大小:在函数中对内部vector进行操作时,也需要确保其大小,否则可能会导致访问越界。
4. 使用迭代器进行遍历:可以使用迭代器对vector<vector<string>>中的元素进行遍历,这样可以避免访问越界和复制数组的开销。
5. 使用const关键字:如果不需要在函数中修改vector<vector<string>>的值,可以使用const关键字来保证其不被修改。
综上所述,使用vector<vector<string>>作为函数参数时需要注意一些细节,以确保程序的正确性和效率。
vector<int> 转 vector<string>
您可以使用标准库中的 `std::transform` 函数来将一个 `vector<int>` 转换为 `vector<string>`。下面是一个示例代码:
```cpp
#include <iostream>
#include <vector>
#include <string>
#include <algorithm>
int main() {
std::vector<int> intVector = {1, 2, 3, 4, 5};
// 创建一个空的 vector<string>
std::vector<std::string> stringVector(intVector.size());
// 使用 std::transform 进行转换
std::transform(intVector.begin(), intVector.end(), stringVector.begin(), [](int num) {
return std::to_string(num);
});
// 打印转换后的 vector<string>
for (const auto& str : stringVector) {
std::cout << str << " ";
}
std::cout << std::endl;
return 0;
}
```
输出结果将会是:`1 2 3 4 5`,每个整数都转换成了字符串。
相关推荐
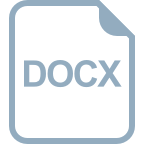
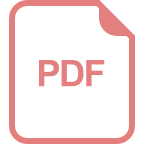












