波士顿房价预测python
时间: 2023-09-03 16:15:14 浏览: 89
这是一个典型的机器学习问题,可以使用Python中的许多库和算法来解决。以下是一个简单的波士顿房价预测Python示例:
1. 导入所需的库和数据集:
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.datasets import load_boston
boston = load_boston()
```
2. 将数据集转换为pandas dataframe,并查看其结构:
```python
boston_df = pd.DataFrame(boston.data, columns=boston.feature_names)
boston_df['MEDV'] = boston.target
print(boston_df.head())
```
3. 对数据集进行可视化分析:
```python
plt.scatter(boston_df['RM'], boston_df['MEDV'], alpha=0.3)
plt.xlabel('Average number of rooms per dwelling (RM)')
plt.ylabel('Median value of owner-occupied homes in $1000s (MEDV)')
plt.show()
```
4. 划分数据集为训练集和测试集:
```python
from sklearn.model_selection import train_test_split
X = boston_df.drop('MEDV', axis=1)
y = boston_df['MEDV']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=1)
```
5. 使用线性回归模型进行训练和预测:
```python
from sklearn.linear_model import LinearRegression
lr = LinearRegression()
lr.fit(X_train, y_train)
y_pred = lr.predict(X_test)
```
6. 评估模型的性能:
```python
from sklearn.metrics import mean_squared_error, r2_score
print('Mean Squared Error: %.2f' % mean_squared_error(y_test, y_pred))
print('Coefficient of determination (R^2): %.2f' % r2_score(y_test, y_pred))
```
7. 可选地,使用交叉验证进行模型评估:
```python
from sklearn.model_selection import cross_val_score
scores = cross_val_score(lr, X, y, cv=5)
print('Cross-validation scores: ', scores)
print('Average cross-validation score: ', np.mean(scores))
```
这是一个简单的波士顿房价预测Python示例,可以根据需要进行修改和扩展。
相关推荐
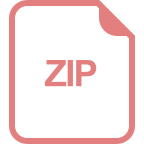











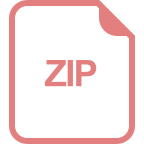