python 使用百度翻译将整个英文网页翻译成中文网页代码示例
时间: 2023-07-15 07:14:04 浏览: 54
以下是使用 Python 3 和百度翻译API将整个英文网页翻译成中文网页的示例代码:
```python
import requests
import hashlib
import random
import json
# 百度翻译API的信息
app_id = 'your_app_id'
secret_key = 'your_secret_key'
# 要翻译的英文网页的URL
url = 'https://www.example.com'
# 获取网页内容
response = requests.get(url)
html_content = response.content.decode('utf-8')
# 使用百度翻译API进行翻译
salt = random.randint(32768, 65536)
sign = app_id + html_content + str(salt) + secret_key
sign = hashlib.md5(sign.encode('utf-8')).hexdigest()
api_url = 'http://api.fanyi.baidu.com/api/trans/vip/translate'
params = {
'q': html_content,
'from': 'en',
'to': 'zh',
'appid': app_id,
'salt': salt,
'sign': sign
}
response = requests.get(api_url, params=params)
result = json.loads(response.text)
# 将翻译结果替换原始的英文网页内容
translated_content = result['trans_result'][0]['dst']
html_content = html_content.replace(html_content, translated_content)
# 将翻译后的内容写入到本地文件中
with open('translated.html', 'w', encoding='utf-8') as f:
f.write(html_content)
```
上述代码中,需要替换掉 `your_app_id` 和 `your_secret_key` 为你的百度翻译API的信息。同时,需要安装 `requests` 库来发送网络请求和 `hashlib` 库来进行MD5加密。此外,还需要通过 `json` 库来解析API的响应。最后,将翻译后的网页内容写入到本地文件 `translated.html` 中。
相关推荐
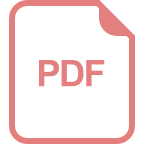
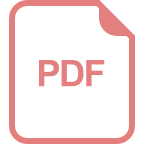














