生成一段CIFAR10_CNN_weights.h5和.pth文件转换的代码
时间: 2024-02-13 19:01:52 浏览: 30
以下是一个将CIFAR10_CNN_weights.h5文件转换为CIFAR10_CNN_weights.pth文件的示例代码:
```python
import torch
import h5py
# 加载h5文件中的权重参数
with h5py.File('CIFAR10_CNN_weights.h5', 'r') as f:
conv1_weight = torch.Tensor(f['conv1/conv1_W:0'][:])
conv1_bias = torch.Tensor(f['conv1/conv1_b:0'][:])
conv2_weight = torch.Tensor(f['conv2/conv2_W:0'][:])
conv2_bias = torch.Tensor(f['conv2/conv2_b:0'][:])
fc1_weight = torch.Tensor(f['fc1/fc1_W:0'][:])
fc1_bias = torch.Tensor(f['fc1/fc1_b:0'][:])
fc2_weight = torch.Tensor(f['fc2/fc2_W:0'][:])
fc2_bias = torch.Tensor(f['fc2/fc2_b:0'][:])
# 创建PyTorch模型
model = torch.nn.Sequential(
torch.nn.Conv2d(in_channels=3, out_channels=32, kernel_size=3, padding=1),
torch.nn.ReLU(),
torch.nn.MaxPool2d(kernel_size=2),
torch.nn.Conv2d(in_channels=32, out_channels=64, kernel_size=3, padding=1),
torch.nn.ReLU(),
torch.nn.MaxPool2d(kernel_size=2),
torch.nn.Flatten(),
torch.nn.Linear(in_features=64 * 8 * 8, out_features=512),
torch.nn.ReLU(),
torch.nn.Linear(in_features=512, out_features=10)
)
# 将权重参数加载到PyTorch模型中
model[0].weight.data = conv1_weight.permute(3, 2, 0, 1)
model[0].bias.data = conv1_bias
model[3].weight.data = conv2_weight.permute(3, 2, 0, 1)
model[3].bias.data = conv2_bias
model[6].weight.data = fc1_weight.t()
model[6].bias.data = fc1_bias
model[9].weight.data = fc2_weight.t()
model[9].bias.data = fc2_bias
# 保存权重参数为.pth文件
torch.save(model.state_dict(), 'CIFAR10_CNN_weights.pth')
```
需要注意的是,在转换过程中需要根据模型结构和权重参数的命名规则来获取相应的权重参数,同时需要注意权重参数的形状和数据类型。
相关推荐
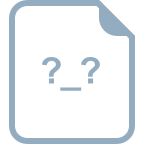














