sm4-ecb加密工具类
时间: 2023-10-31 13:58:28 浏览: 96
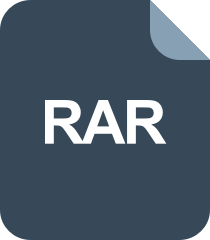
KYSharp.SM_code.rar
以下是一个使用Java语言实现的SM4-ECB加密工具类,供参考:
```java
import org.bouncycastle.crypto.engines.SM4Engine;
import org.bouncycastle.crypto.modes.ECBBlockCipher;
import org.bouncycastle.crypto.params.KeyParameter;
import org.bouncycastle.util.encoders.Hex;
public class SM4Util {
/**
* SM4 ECB加密
*
* @param key 加密密钥,长度为16字节
* @param data 待加密数据
* @return 加密结果,16进制字符串
*/
public static String encryptECB(String key, String data) {
try {
byte[] keyBytes = Hex.decode(key);
byte[] dataBytes = Hex.decode(data);
// 初始化SM4引擎
SM4Engine engine = new SM4Engine();
ECBBlockCipher cipher = new ECBBlockCipher(engine);
cipher.init(true, new KeyParameter(keyBytes));
// 加密
byte[] out = new byte[dataBytes.length];
int len = cipher.processBlock(dataBytes, 0, dataBytes.length, out, 0);
cipher.doFinal(out, len);
// 转换为16进制字符串
return Hex.toHexString(out);
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
/**
* SM4 ECB解密
*
* @param key 解密密钥,长度为16字节
* @param data 待解密数据,16进制字符串
* @return 解密结果
*/
public static String decryptECB(String key, String data) {
try {
byte[] keyBytes = Hex.decode(key);
byte[] dataBytes = Hex.decode(data);
// 初始化SM4引擎
SM4Engine engine = new SM4Engine();
ECBBlockCipher cipher = new ECBBlockCipher(engine);
cipher.init(false, new KeyParameter(keyBytes));
// 解密
byte[] out = new byte[dataBytes.length];
int len = cipher.processBlock(dataBytes, 0, dataBytes.length, out, 0);
cipher.doFinal(out, len);
// 转换为字符串
return new String(out, "UTF-8");
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
```
使用示例:
```java
public class SM4Test {
public static void main(String[] args) {
String key = "0123456789abcdef0123456789abcdef"; // 16字节密钥
String data = "hello world!";
String encrypted = SM4Util.encryptECB(key, data);
String decrypted = SM4Util.decryptECB(key, encrypted);
System.out.println("加密前:" + data);
System.out.println("加密后:" + encrypted);
System.out.println("解密后:" + decrypted);
}
}
```
需要注意的是,以上代码使用了Bouncy Castle库提供的SM4实现。在使用之前需要先添加Bouncy Castle的依赖。
阅读全文
相关推荐
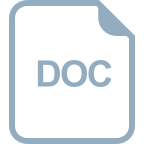
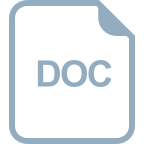
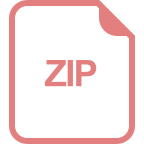
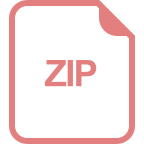
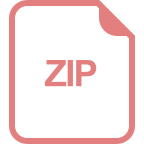
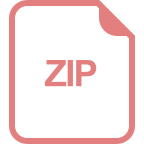
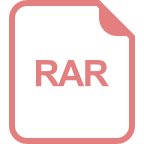
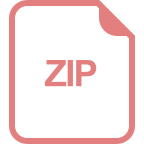
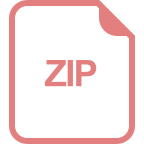
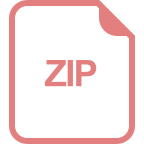




