vuex是怎么获取到里面的值
时间: 2024-04-23 11:24:55 浏览: 14
在 Vuex 中,可以通过 `this.$store.state` 来访问 state 中的值。例如,如果有一个名为 `count` 的 state,可以通过 `this.$store.state.count` 来获取它的值。
除了直接访问 state 外,还可以通过 getters 来获取 state 中的值。getters 可以看作是对 state 的计算属性,可以接受 state 作为参数,并返回一个计算后的值。在组件中使用 getters 时,可以通过 `this.$store.getters.getterName` 来获取计算后的值。其中,`getterName` 是 getters 中定义的函数名。
另外,在 Vuex 中,还可以通过 mutations 和 actions 来修改 state 中的值。mutations 是同步修改 state 的方法,而 actions 是异步修改 state 的方法。在组件中调用 mutations 和 actions 的方式都是通过 `this.$store.commit('mutationName')` 和 `this.$store.dispatch('actionName')` 来触发相应的方法。其中,`mutationName` 和 `actionName` 分别是 mutations 和 actions 中定义的方法名。
相关问题
模块化vuex怎么获取模块里面的值
在使用模块化的 Vuex 中,获取模块里面的值需要使用模块命名空间(module namespace)。
在定义模块时,可以通过 `namespaced: true` 开启模块命名空间,这样在获取模块内部的状态、getters、mutations、actions 时,就需要加上模块的命名空间。
举个例子,假设我们有一个 `user` 模块,其中定义了一个 `state`:
```javascript
// user.js
export default {
namespaced: true,
state: {
name: '小明',
age: 18
}
}
```
如果要在另一个模块中获取 `user` 模块的 `name` 值,可以这样写:
```javascript
// other.js
export default {
computed: {
userName() {
return this.$store.state.user.name
}
}
}
```
但是在使用模块命名空间后,就需要添加模块命名空间前缀:
```javascript
// user.js
export default {
namespaced: true,
state: {
name: '小明',
age: 18
}
}
// other.js
export default {
computed: {
userName() {
return this.$store.state.user.name // 错误,需要加上模块命名空间前缀
// return this.$store.state['user'].name // 正确的方式
}
}
}
```
如果需要在 getters、mutations、actions 中访问模块内部的状态和其他函数,也需要加上模块命名空间前缀,例如:
```javascript
// user.js
export default {
namespaced: true,
state: {
name: '小明',
age: 18
},
getters: {
getNameAndAge(state) {
return `${state.name} - ${state.age}`
}
},
mutations: {
setName(state, newName) {
state.name = newName
}
},
actions: {
changeName({ commit }, newName) {
commit('setName', newName)
}
}
}
// other.js
export default {
computed: {
nameAndAge() {
return this.$store.getters['user/getNameAndAge'] // 获取 user 模块的 getters
}
},
methods: {
changeName(newName) {
this.$store.commit('user/setName', newName) // 调用 user 模块的 mutation
this.$store.dispatch('user/changeName', newName) // 调用 user 模块的 action
}
}
}
```
以上就是在模块化 Vuex 中获取模块里面的值的方法。
vuex如何获取state里面的数据
在Vuex中,你可以通过使用`this.$store.state`来访问state中的数据。具体来说,你可以使用`this.$store.state.propertyName`来获取state中的特定属性值。
例如,假设你的state对象包含一个名为`count`的属性,你可以使用以下方式获取它的值:
```javascript
const count = this.$store.state.count;
```
请注意,这段代码应该在Vue组件的方法中使用,因为`this.$store`只在Vue组件实例中可用。
当然,你也可以使用Vuex提供的辅助函数`mapState`来简化代码。使用`mapState`,你可以将state中的属性映射到组件的计算属性中。
首先,在你的组件中导入`mapState`函数:
```javascript
import { mapState } from 'vuex';
```
然后,在计算属性中使用`mapState`函数将state属性映射到组件:
```javascript
computed: {
...mapState(['count'])
}
```
现在,你可以直接在组件中使用`count`属性来访问state中的`count`值。
这就是在Vuex中获取state数据的方法。希望对你有所帮助!如果你有任何其他问题,请随时提问。
相关推荐
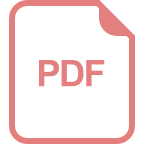












