vuex怎么改变state里面的值
时间: 2023-02-07 18:41:02 浏览: 117
在 Vuex 中,你可以通过提交 mutation 来改变 state 中的值。
首先,你需要在 store 中定义 mutation:
```
const store = new Vuex.Store({
state: {
count: 0
},
mutations: {
increment (state) {
// 变更状态
state.count++
}
}
})
```
然后,你可以在组件中使用 `this.$store.commit('increment')` 来提交 mutation:
```
methods: {
increment () {
this.$store.commit('increment')
}
}
```
你也可以在 mutation 中传入额外的参数,例如:
```
mutations: {
increment (state, n) {
state.count += n
}
}
// 提交 mutation
this.$store.commit('increment', 10)
```
注意:你不应该直接改变 state 中的值,而是应该通过提交 mutation 来改变。这样可以使得你的代码更易于调试和测试。
相关问题
vuex如何获取state里面的数据
在使用 Vuex 进行状态管理的项目中,你可以通过以下方式获取 `state` 中的数据:
1. 在组件内部通过 `this.$store.state` 来访问整个 `state` 对象,然后通过属性访问的方式获取具体的数据。例如,如果你的 `state` 对象中有一个名为 `count` 的属性,你可以使用 `this.$store.state.count` 来获取它的值。
2. 可以使用 `mapState` 辅助函数将 `state` 映射为组件的计算属性。在组件中引入 `mapState` 函数后,你可以将需要的 `state` 属性映射为组件的计算属性,这样就可以直接在组件中使用这些属性了。例如:
```javascript
import { mapState } from 'vuex';
export default {
// ...
computed: {
...mapState(['count']) // 将 state 中的 count 属性映射为组件的计算属性
}
}
```
然后在组件中就可以使用 `this.count` 来获取 `state` 中的数据。
3. 如果你需要对 `state` 中的数据进行一些处理或计算,你也可以将这些处理逻辑放在 Vuex 的 `getters` 中,并通过 `this.$store.getters` 来获取处理后的数据。例如,在 Vuex 的 `getters` 中定义一个名为 `doubleCount` 的 getter:
```javascript
// ...
getters: {
doubleCount: state => state.count * 2
}
```
然后在组件中你可以通过 `this.$store.getters.doubleCount` 来获取处理后的数据。
希望能够帮助到你!如果还有其他问题,请继续提问。
vuex组件里怎么改变值
在 Vuex 中,我们需要使用 mutations 来改变 state 中的值。mutations 是一个包含多个函数的对象,每个函数都有一个 state 参数,你可以在函数中修改 state 的值。在组件中,你可以使用 this.$store.commit('mutation的名称') 来调用 mutations 中的函数。
例如,如果你想要在组件中改变 state 中的 count 值,你可以创建一个名为 increment 的 mutation:
```
// Vuex store 中的 mutations
const mutations = {
increment(state) {
state.count++
}
}
```
然后在组件中调用:
```
// 组件中
methods: {
increment() {
this.$store.commit('increment')
}
}
```
这样就可以通过点击按钮来触发 increment 函数,从而改变 state 中的 count 值。
阅读全文
相关推荐
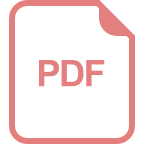
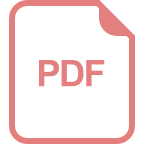
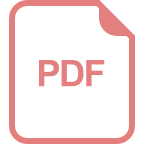

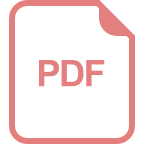
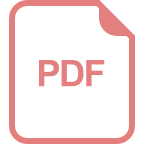
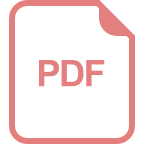
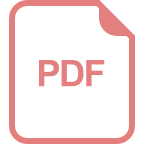
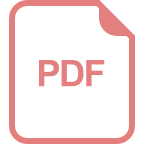
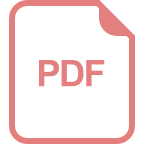
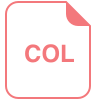




