编写程序,统计文件中大写字母、小写字母、数字字符以及其他字符的出现次数。统计完依次输出大写字母、小写字母、数字字符、其他字符的出现次数,中间用半角空格分隔
时间: 2023-10-26 21:05:06 浏览: 77
可以使用Python编写一个统计文件中字符出现次数的程序:
```python
filename = input("请输入文件名:")
upper_count = 0
lower_count = 0
digit_count = 0
other_count = 0
with open(filename, 'r') as f:
for line in f:
for char in line:
if char.isupper():
upper_count += 1
elif char.islower():
lower_count += 1
elif char.isdigit():
digit_count += 1
else:
other_count += 1
print(upper_count, lower_count, digit_count, other_count)
```
程序中使用`input`函数获取用户输入的文件名,然后依次遍历文件中的每一个字符,使用`isupper`、`islower`和`isdigit`方法判断字符的类型,从而统计出现次数。最后使用`print`函数输出结果。
相关问题
编写程序,统计文件“in.txt”中大写字母、小写字母、数字字符以及其他字符的出现次数。
好的,这个问题我可以回答。以下是 Python 代码实现:
```python
upper_count = 0
lower_count = 0
digit_count = 0
other_count = 0
with open("in.txt", "r") as file:
data = file.read()
for char in data:
if char.isupper():
upper_count += 1
elif char.islower():
lower_count += 1
elif char.isdigit():
digit_count += 1
else:
other_count += 1
print(f"大写字母出现次数:{upper_count}")
print(f"小写字母出现次数:{lower_count}")
print(f"数字字符出现次数:{digit_count}")
print(f"其他字符出现次数:{other_count}")
```
以上代码中,我们首先定义了四个变量来统计大写字母、小写字母、数字字符以及其他字符的出现次数。然后,我们使用 `with open` 语句来打开文件,并读取文件内容到 `data` 变量中。接着,我们遍历每个字符,通过 `isupper()`、`islower()` 和 `isdigit()` 方法来判断字符是大写字母、小写字母还是数字字符,从而更新对应的计数器。最后,我们输出每个计数器的值,即为各种字符出现的次数。
Python中编写程序,统计文件“in.txt”中大写字母、小写字母、数字字符以及其他字符的出现次数
可以使用Python中的文件操作和字符串操作来实现这个功能。具体步骤如下:
1. 打开文件,读取文件内容,并关闭文件。
2. 遍历文件中的每一个字符,判断它属于哪一类字符(大写字母、小写字母、数字字符或其他字符),并在相应的计数器中加1。
3. 输出各类字符出现的次数。
下面是代码实现:
```python
# 打开文件,读取文件内容并关闭文件
with open('in.txt', 'r') as f:
content = f.read()
# 初始化计数器
upper_count = 0
lower_count = 0
digit_count = 0
other_count = 0
# 遍历文件中的每一个字符,判断它属于哪一类字符,并在相应的计数器中加1
for c in content:
if c.isupper():
upper_count += 1
elif c.islower():
lower_count += 1
elif c.isdigit():
digit_count += 1
else:
other_count += 1
# 输出各类字符出现的次数
print("大写字母出现次数:", upper_count)
print("小写字母出现次数:", lower_count)
print("数字字符出现次数:", digit_count)
print("其他字符出现次数:", other_count)
```
注意,这里的文件名是“in.txt”,如果你的文件名不同,请做相应修改。
相关推荐
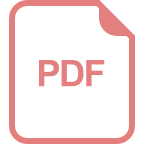
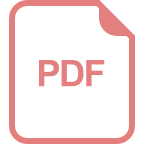
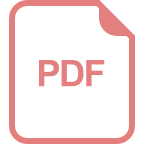












