用springboot写调用带token的api接口的程序
时间: 2024-06-10 22:06:50 浏览: 23
很高兴为您解答问题。关于使用SpringBoot编写调用带Token的API接口的程序,您可以采用JWT(JSON Web Token)来实现身份验证。JWT是一种开放标准,用于在各方之间安全地传输信息,可以包含用户身份信息、过期时间等。您可以通过在请求头部添加Token信息来进行身份验证,防止未经授权的访问。建议您阅读相关文档,深入了解JWT的使用。
相关问题
springboot调用小程序API弹框消息提醒
你可以通过调用小程序的API来实现弹框消息提醒。具体步骤如下:
1. 在小程序后台获取 access_token,用于后续接口调用。可以使用微信提供的 API:https://developers.weixin.qq.com/miniprogram/dev/api-backend/open-api/access-token/auth.getAccessToken.html
2. 调用小程序的订阅消息接口,发送订阅消息给用户。具体接口为:https://developers.weixin.qq.com/miniprogram/dev/api-backend/open-api/subscribe-message/subscribeMessage.send.html
3. 在 Spring Boot 中发送 HTTP 请求调用上述接口。你可以使用 Java 的 HTTP 请求库,例如 Apache HttpClient 或者 OkHttp。
以下是一个简单的示例代码:
```java
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
public class WeChatApiUtils {
public static void sendSubscribeMessage(String accessToken, String openId, String templateId, String message) throws IOException {
String url = "https://api.weixin.qq.com/cgi-bin/message/subscribe/send?access_token=" + accessToken;
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(url);
// 构造请求体
String requestBody = "{\"touser\":\"" + openId + "\",\"template_id\":\"" + templateId + "\",\"data\":{\"message\":{\"value\":\"" + message + "\"}}}";
StringEntity requestEntity = new StringEntity(requestBody, ContentType.APPLICATION_JSON);
httpPost.setEntity(requestEntity);
// 发送请求并获取响应
HttpResponse response = httpClient.execute(httpPost);
HttpEntity responseEntity = response.getEntity();
String responseBody = EntityUtils.toString(responseEntity);
System.out.println(responseBody); // 这里可以根据实际需求处理响应结果
}
}
```
你需要替换其中的 accessToken、openId、templateId 和 message 参数为实际的值。其中,accessToken 是第一步获取到的访问令牌,openId 是用户的唯一标识,templateId 是订阅消息的模板 ID,message 是要发送的消息内容。
请注意,为了保证代码的安全性和可靠性,建议在生产环境中使用合适的加密和身份验证机制。此外,你需要根据具体的业务需求和小程序的订阅消息接口文档进行参数的调整和接口调用的逻辑处理。
用springboot写一个登录的接口
好的,以下是一个用Spring Boot编写的简单登录接口的示例:
首先,需要添加Spring Security和Spring Web依赖。在Maven项目中,可以在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
然后,创建一个用于验证用户凭据的服务类,例如:
```java
@Service
public class AuthenticationService {
public boolean authenticate(String username, String password) {
// 进行身份验证逻辑,比如查询数据库或调用外部API
// 如果验证成功,返回true,否则返回false
}
}
```
接下来,创建一个控制器类,用于处理登录请求和返回响应。例如:
```java
@RestController
public class LoginController {
@Autowired
private AuthenticationService authenticationService;
@PostMapping("/login")
public ResponseEntity<?> login(@RequestBody LoginForm loginForm) {
boolean success = authenticationService.authenticate(loginForm.getUsername(), loginForm.getPassword());
if (success) {
// 如果验证成功,返回一个JWT令牌
String token = // 生成JWT令牌的逻辑
return ResponseEntity.ok(new LoginResponse(token));
} else {
// 如果验证失败,返回一个401未授权响应
return ResponseEntity.status(HttpStatus.UNAUTHORIZED).build();
}
}
// 定义一个用于接收登录请求的表单类
static class LoginForm {
private String username;
private String password;
// 省略getter和setter
}
// 定义一个用于返回登录响应的类
static class LoginResponse {
private String token;
public LoginResponse(String token) {
this.token = token;
}
// 省略getter和setter
}
}
```
在上面的示例中,我们使用了一个简单的JWT令牌来表示用户已经通过身份验证。在实际生产环境中,您可能需要使用更复杂的令牌方案,比如OAuth2。
最后,启动Spring Boot应用程序并访问/login端点即可进行登录。例如,您可以使用cURL发送一个POST请求:
```
curl -X POST \
http://localhost:8080/login \
-H 'Content-Type: application/json' \
-d '{
"username": "alice",
"password": "password123"
}'
```
注意,这只是一个简单的示例,并不包含所有必要的安全措施。在生产环境中,您需要更仔细地考虑安全性,并采取其他措施来保护您的应用程序。
相关推荐
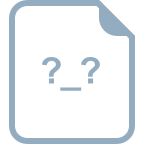
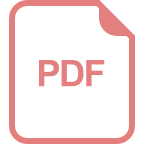
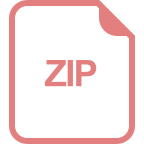












