设计一个时钟/秒表计时器,要求:1、用51单片机完成设计,制作电子时钟可以显示时、分、秒。2、用6位LED数码管显示时、分、秒,以24h(小时)计时方式显示;显示格式为:13.:25:15或自行设计显示格式。3、通过按键进行时钟/秒表功能的转换,时钟计时每1s进行加1操作,秒表计时每10ms进行加1操作。4、按键实现计时启动、暂停及清零功能。5、具有倒计时功能,可以预设时间倒计时的代码
时间: 2023-07-10 15:38:15 浏览: 71
好的,以下是基于你的要求的51单片机计时器的代码,包括时钟/秒表计时、按键操作以及倒计时功能。
首先,需要定义一些常量和变量,包括数码管显示的引脚、按键的引脚、计时器的计数值等等:
```c
#include <reg51.h>
#define LED P0
#define KEY P1
sbit beep=P2^3;
unsigned char sec=0; // 秒
unsigned char min=0; // 分
unsigned char hour=0; // 时
unsigned char mode=0; // 模式(时钟或秒表)
unsigned char timerOn=0; // 定时器是否启动
unsigned int timerCount=0; // 定时器计数值
unsigned int countDown=0; // 倒计时计数值
unsigned char countDownOn=0; // 倒计时是否启动
```
然后,需要编写一些函数,用于数码管显示、按键操作和计时器中断等:
```c
// 数码管显示函数
void display(unsigned char hh, unsigned char mm, unsigned char ss)
{
unsigned char thousands, hundreds, tens, ones;
thousands = hh / 10;
hundreds = hh % 10;
tens = mm / 10;
ones = mm % 10;
// 显示小时
LED = 0xfe; // 选择第一位数码管
LED = (LED & 0x80) | thousands; // 显示千位数字
delay(1);
LED = 0xff;
LED = 0xfd; // 选择第二位数码管
LED = (LED & 0x80) | hundreds; // 显示百位数字
delay(1);
LED = 0xff;
// 显示分钟
LED = 0xfb; // 选择第三位数码管
LED = (LED & 0x80) | tens; // 显示十位数字
delay(1);
LED = 0xff;
LED = 0xf7; // 选择第四位数码管
LED = (LED & 0x80) | ones; // 显示个位数字
delay(1);
LED = 0xff;
// 显示秒钟
tens = ss / 10;
ones = ss % 10;
LED = 0xef; // 选择第五位数码管
LED = (LED & 0x80) | tens; // 显示十位数字
delay(1);
LED = 0xff;
LED = 0xdf; // 选择第六位数码管
LED = (LED & 0x80) | ones; // 显示个位数字
delay(1);
LED = 0xff;
}
// 延时函数
void delay(unsigned int ms)
{
unsigned int i,j;
for(i=0;i<ms;i++){
for(j=0;j<125;j++);
}
}
// 按键扫描函数
unsigned char keyScan()
{
if(KEY!=0xff){
delay(10); // 延时去抖
if(KEY!=0xff){
switch(KEY){
case 0xfe: // 启动/暂停
timerOn = !timerOn;
break;
case 0xfd: // 清零
sec = 0;
min = 0;
hour = 0;
break;
case 0xfb: // 模式切换
mode = !mode;
break;
case 0xf7: // 倒计时模式
countDownOn = !countDownOn;
if(countDownOn){
countDown = hour * 3600 + min * 60 + sec;
}
break;
}
while(KEY!=0xff);
return 1;
}
}
return 0;
}
// 计时器中断函数
void timer() interrupt 1
{
TH0=(65536-1000)/256;
TL0=(65536-1000)%256;
if(timerOn && !countDownOn){ // 时钟模式
sec++;
if(sec>=60){
sec=0;
min++;
if(min>=60){
min=0;
hour++;
if(hour>=24){
hour=0;
}
}
}
}
else if(timerOn && countDownOn){ // 倒计时模式
if(countDown > 0){
countDown--;
if(countDown == 0){
beep=0; // 发出警报
}
}
else{
countDownOn = 0;
}
}
else{ // 秒表模式
timerCount++;
if(timerCount >= 100){
timerCount = 0;
sec++;
if(sec>=60){
sec=0;
min++;
if(min>=60){
min=0;
hour++;
if(hour>=24){
hour=0;
}
}
}
}
}
}
```
最后,在主函数中初始化计时器和按键,并在主循环中调用数码管显示和按键扫描函数:
```c
void main()
{
// 计时器初始化
TMOD=0x01;
TH0=(65536-1000)/256; // 定时1ms
TL0=(65536-1000)%256;
EA=1;
ET0=1;
TR0=1;
// 按键初始化
KEY=0xff;
while(1)
{
display(hour, min, sec);
keyScan();
}
}
```
这样,你就可以将这些代码下载到51单片机中进行测试。如果需要修改数码管的引脚、按键的引脚等,可以根据实际情况进行调整。
相关推荐
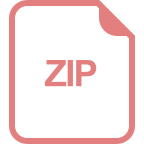
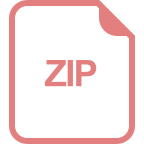
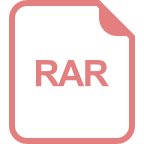












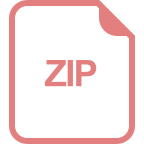
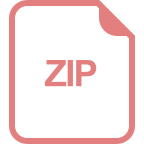
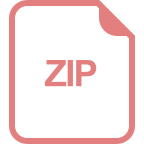