混沌加密算法python_基于混沌Logistic加密算法的图片加密与还原
时间: 2023-12-21 17:06:57 浏览: 301
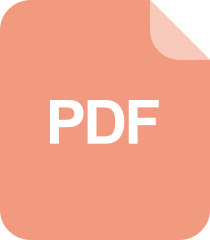
基于Logistic系统的图像模块混沌加密解密算法

混沌加密算法是一种基于混沌现象的加密技术,其加密过程具有高度的随机性和不可预测性,可以有效地保护信息安全。下面介绍一下基于混沌Logistic加密算法的图片加密与还原的实现方法。
首先,需要安装相关的Python库,包括numpy、matplotlib和pillow。可以使用以下命令进行安装:
```
pip install numpy matplotlib pillow
```
加密过程如下:
1. 读入待加密的图片,并将其转换成二进制序列。
2. 生成一个随机的密钥,作为混沌算法的种子。
3. 使用Logistic混沌算法生成一组伪随机数序列。
4. 将伪随机数序列与原始图片的二进制序列进行异或运算,得到密文序列。
5. 将密文序列保存到文件中,并将密钥保存到文件中。
还原过程如下:
1. 读入密文文件和密钥文件。
2. 使用密钥作为Logistic混沌算法的种子,生成一组伪随机数序列。
3. 将伪随机数序列与密文序列进行异或运算,得到原始图片的二进制序列。
4. 将二进制序列转换成图片,保存到文件中。
下面是基于Python实现的代码。
```python
import numpy as np
import matplotlib.pyplot as plt
from PIL import Image
# 定义Logistic混沌函数
def logistic(x, r):
return r * x * (1 - x)
# 生成伪随机数序列
def generate_random_sequence(seed, size):
x = seed
sequence = []
for i in range(size):
x = logistic(x, 3.9)
sequence.append(x)
return sequence
# 加密函数
def encrypt_image(image_file, key_file, encrypted_file):
# 读入图片并转换成二进制序列
image = Image.open(image_file)
image_array = np.array(image)
binary_array = np.unpackbits(image_array)
# 生成密钥
key = np.random.randint(256, size=1)[0]
with open(key_file, 'w') as f:
f.write(str(key))
# 生成伪随机数序列
random_sequence = generate_random_sequence(key, len(binary_array))
# 将伪随机数序列与二进制序列进行异或运算
encrypted_array = np.bitwise_xor(binary_array, np.array(random_sequence)*255)
# 将密文序列保存到文件中
encrypted_image_array = np.packbits(encrypted_array)
encrypted_image = Image.fromarray(encrypted_image_array)
encrypted_image.save(encrypted_file)
# 解密函数
def decrypt_image(encrypted_file, key_file, decrypted_file):
# 读入密文文件和密钥文件
with open(key_file, 'r') as f:
key = int(f.read())
encrypted_image = Image.open(encrypted_file)
encrypted_image_array = np.array(encrypted_image)
binary_array = np.unpackbits(encrypted_image_array)
# 生成伪随机数序列
random_sequence = generate_random_sequence(key, len(binary_array))
# 将伪随机数序列与密文序列进行异或运算
decrypted_array = np.bitwise_xor(binary_array, np.array(random_sequence)*255)
# 将二进制序列转换成图片并保存到文件中
decrypted_image_array = np.packbits(decrypted_array)
decrypted_image = Image.fromarray(decrypted_image_array)
decrypted_image.save(decrypted_file)
# 加密并保存图片
encrypt_image('image.jpg', 'key.txt', 'encrypted_image.jpg')
# 解密并保存图片
decrypt_image('encrypted_image.jpg', 'key.txt', 'decrypted_image.jpg')
```
需要注意的是,由于Logistic混沌算法对初始种子非常敏感,因此生成的伪随机数序列可能会有很大的偏差。为了避免这种情况,可以通过多次运行算法,选择最优的种子作为密钥。
阅读全文
相关推荐
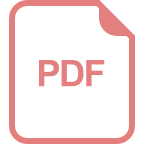
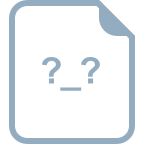
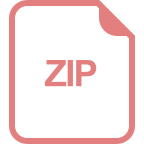
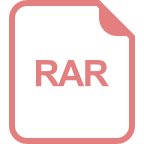
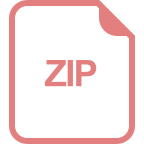
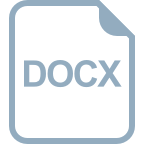
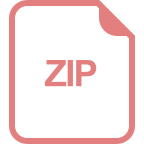
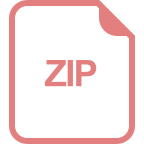
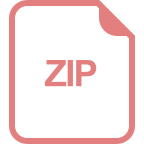
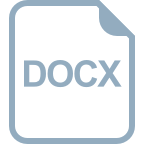
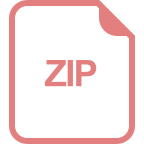
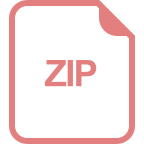
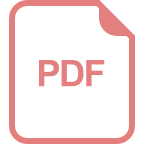
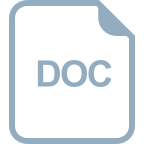
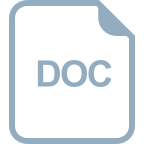
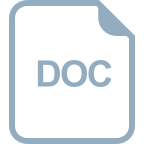
