python 动态正弦曲线,利用pyside2的信号和槽从文本块获取幅值和频率
时间: 2023-05-17 19:05:35 浏览: 97
可以使用Python的matplotlib库来绘制动态正弦曲线,同时利用pyside2的信号和槽从文本块获取幅值和频率。以下是一个简单的示例代码:
```python
import sys
import numpy as np
from matplotlib.backends.qt_compat import QtCore, QtWidgets, is_pyqt5
from matplotlib.figure import Figure
from matplotlib.backends.backend_qt5agg import FigureCanvasQTAgg as FigureCanvas
if is_pyqt5():
from PyQt5.QtCore import pyqtSignal as Signal
else:
from PyQt4.QtCore import pyqtSignal as Signal
class DynamicSinusoid(QtWidgets.QWidget):
def __init__(self, parent=None):
super(DynamicSinusoid, self).__init__(parent)
# Create a figure and a canvas
self.figure = Figure()
self.canvas = FigureCanvas(self.figure)
# Create a vertical box layout
layout = QtWidgets.QVBoxLayout()
layout.addWidget(self.canvas)
self.setLayout(layout)
# Create a timer for updating the plot
self.timer = QtCore.QTimer()
self.timer.timeout.connect(self.update_plot)
# Initialize the plot
self.ax = self.figure.add_subplot(111)
self.ax.set_xlim(0, 2*np.pi)
self.ax.set_ylim(-1, 1)
self.ax.set_xlabel('Time')
self.ax.set_ylabel('Amplitude')
self.line, = self.ax.plot([], [])
# Create a text block for entering the amplitude and frequency
self.amplitude_edit = QtWidgets.QLineEdit()
self.frequency_edit = QtWidgets.QLineEdit()
self.amplitude_edit.setValidator(QtGui.QDoubleValidator())
self.frequency_edit.setValidator(QtGui.QDoubleValidator())
self.amplitude_edit.textChanged.connect(self.update_amplitude)
self.frequency_edit.textChanged.connect(self.update_frequency)
# Add the text block to the layout
layout.addWidget(self.amplitude_edit)
layout.addWidget(self.frequency_edit)
# Start the timer
self.timer.start(50)
# Initialize the amplitude and frequency
self.amplitude = 1.0
self.frequency = 1.0
def update_plot(self):
# Generate the data for the plot
t = np.linspace(0, 2*np.pi, 1000)
y = self.amplitude * np.sin(self.frequency * t)
# Update the plot
self.line.set_data(t, y)
self.canvas.draw()
def update_amplitude(self, text):
# Update the amplitude
try:
self.amplitude = float(text)
except ValueError:
pass
def update_frequency(self, text):
# Update the frequency
try:
self.frequency = float(text)
except ValueError:
pass
if __name__ == '__main__':
app = QtWidgets.QApplication(sys.argv)
widget = DynamicSinusoid()
widget.show()
sys.exit(app.exec_())
```
在这个示例中,我们创建了一个名为DynamicSinusoid的QWidget子类,它包含一个matplotlib图形和两个文本块,用于输入幅值和频率。我们使用一个定时器来更新图形,每50毫秒更新一次。在update_plot方法中,我们生成正弦波的数据,并使用set_data方法将数据设置为matplotlib线对象的数据。在update_amplitude和update_frequency方法中,我们将文本块中输入的值转换为浮点数,并将其存储在实例变量中,以便在下一次更新图形时使用。
要运行这个示例,只需运行python脚本即可。在文本块中输入幅值和频率,然后观察动态正弦曲线的变化。
相关推荐
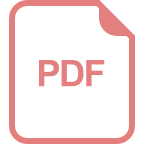














