python 中grid如何设置宽高 排版
时间: 2023-09-25 18:13:09 浏览: 161
在 Python 中使用 grid 进行排版时,可以通过设置 rowconfigure 和 columnconfigure 方法来设置行和列的宽度和高度。具体的代码如下:
```python
import tkinter as tk
root = tk.Tk()
# 设置行和列的宽度和高度
root.rowconfigure(0, minsize=50)
root.columnconfigure(0, minsize=50)
# 创建两个标签并使用 grid 进行排版
label1 = tk.Label(root, text="Label 1", bg="red")
label1.grid(row=0, column=0, padx=5, pady=5)
label2 = tk.Label(root, text="Label 2", bg="blue")
label2.grid(row=1, column=0, padx=5, pady=5)
root.mainloop()
```
在上面的代码中,我们设置了第一行和第一列的最小尺寸为 50,然后创建了两个标签并使用 grid 进行排版。我们还使用 padx 和 pady 参数来设置标签之间的水平和垂直间距。
相关问题
tk用grid排版方式
Tkinter中的`grid`布局管理器是一种二维网格布局方法,用于将控件按照预定义的行和列排列在窗口内。它的基本思想是将控件放在一个由行和列组成的格子中,每个控件占据一个或多个单元格。
以下是一个使用`grid`的例子,展示了如何创建一个简单的窗口并使用`grid`来安排控件:
```python
import tkinter as tk
# 创建主窗口
root = tk.Tk()
root.title("Grid Layout Example")
# 定义一些控件及其位置
label1 = tk.Label(root, text="Label 1")
label2 = tk.Label(root, text="Label 2")
button1 = tk.Button(root, text="Button 1")
button2 = tk.Button(root, text="Button 2")
# 设置控件的尺寸和位置
label1.grid(row=0, column=0) # 第一行第一列
label2.grid(row=0, column=1) # 同一行第二列
button1.grid(row=1, column=0) # 第二行第一列
button2.grid(row=1, column=1) # 第二行第二列
# 运行主循环
root.mainloop()
```
在这个例子中,`row`参数控制垂直方向的位置,`column`参数控制水平方向的位置。如果你想要跨越多列或多行,可以设置`sticky`属性,如`sticky="ew"`会使控件尽可能地填满其所在的行和列。
python 识别图片中的人脸,并裁剪人脸为一寸免冠照片,将背景换为红色,排版成一版8张图片
在Python中,可以使用深度学习库如OpenCV、Dlib和PIL等配合人脸识别技术来实现这个需求。以下是一个简单的步骤概述:
1. **安装必要的库**:
- `dlib`或`face_recognition`用于人脸检测
- `Pillow`或`Image`处理图像转换
2. **人脸检测**:
使用OpenCV的`haarcascade_frontalface_default.xml`预训练模型,或者通过dlib库的`get_frontal_face_detector()`函数获取面部检测器。
```python
import cv2
from dlib import get_frontal_face_detector
detector = get_frontal_face_detector()
```
3. **裁剪人脸**:
找到人脸边界框后,使用`cv2.resize()`函数调整大小为一寸尺寸并保存为单独的图片。
4. **更换背景**:
使用`cv2.rectangle()`绘制矩形框替换背景,这里选择红色背景。
5. **批量处理**:
循环遍历图片文件,对每张图片执行上述操作,生成8张排列好的图片。
6. **排版**:
可能需要使用PIL的`ImageGrid`或自定义逻辑来创建网格布局,将8张人脸图片放在一起。
以下是一个简化的示例代码片段,实际应用中需要完整处理图片路径和文件名等细节:
```python
import cv2
import os
from PIL import Image, ImageDraw, ImageFont, ImageGrid
def process_image(img_path):
img = cv2.imread(img_path)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
faces = detector(gray)
for face in faces:
x, y, w, h = face.left(), face.top(), face.width(), face.height()
cropped_face = img[y:y+h, x:x+w]
resized_face = cv2.resize(cropped_face, (100, 100)) # 一寸尺寸
red_background = np.full((100, 100, 3), (255, 0, 0), dtype=np.uint8) # 红色背景
face_with_red = cv2.addWeighted(resized_face, 0.7, red_background, 0.3, 0)
# 将人脸添加到一个新的Image中(假设你已经处理了8张图片)
combined_img = ... # 你的排版逻辑
# 遍历你的图片目录
image_dir = 'your/image/directory'
images = [os.path.join(image_dir, f) for f in os.listdir(image_dir)]
for i, img in enumerate(images):
if i < 8:
process_image(img)
```
阅读全文
相关推荐
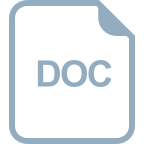
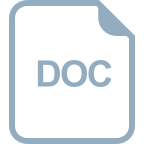
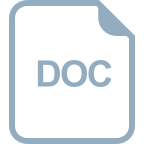
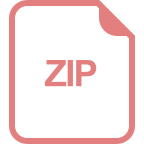
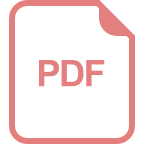
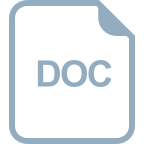
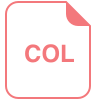
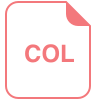
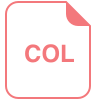
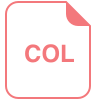
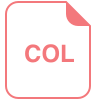
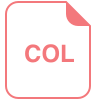
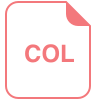
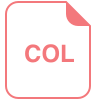


