优化这段代码#include<stdio.h> #include<stdlib.h> #define MAXSIZE 6 //最大长度 typedef int QElemType; typedef struct { QElemType *base; //初始化的动态分配存储空间 int front; int rear; //下标 }SqQueue; enum Status{ERROR,OK}; //循环队列初始化 Status InitQueue(SqQueue &Q) { Q.base=new QElemType[MAXSIZE]; if(!Q.base) return ERROR; Q.front=Q.rear=0; //队空 return OK; } //入队 Status EnQueue(SqQueue &Q,QElemType e) { //添加判断语句,如果rear超过max,则直接将其从a[0]重新开始存储,如果rear+1和front重合,则表示数组已满 if ((Q.rear+1)%MAXSIZE==Q.front) { return ERROR; } Q.base[Q.rear]=e; Q.rear=(Q.rear+1)%MAXSIZE; return OK; } //出队 Status DeQueue(SqQueue &Q,QElemType &e) { //如果front==rear,表示队列为空 if(Q.front==Q.rear) return ERROR; e=Q.base[Q.front]; //front不再直接 +1,而是+1后同max进行比较,如果=max,则直接跳转到 a[0] Q.front=(Q.front+1)%MAXSIZE; return OK; } //循环队列长度 int QueueLength (SqQueue Q) { return (Q.rear-Q.front+MAXSIZE)%MAXSIZE; } int main() { QElemType e; SqQueue Q; InitQueue(Q); printf("开始入队\n"); for(int i=0;i<MAXSIZE-1;i++) { scanf("%d",&e); EnQueue(Q,e); } printf("出一个队列元素:\n"); DeQueue(Q,e); printf("%d \n",e); printf("再入一个元素\n"); scanf("%d",&e); EnQueue(Q,e); printf("全部出队列\n"); for(i=0;i<MAXSIZE-1;i++) { DeQueue(Q,e); printf("%d ",e); } printf("此时循环队列长度为 :%d\n",MAXSIZE-1-QueueLength(Q)); return 0; }
时间: 2024-01-24 15:02:46 浏览: 39
这段代码已经进行了一些优化,但还有一些可以改进的地方:
1. 变量命名
变量名最好能够反映其具体含义,比如将 `Q.base` 改为 `Q.data`,将 `Q.front` 改为 `Q.head`。
2. 枚举类型
枚举类型建议使用驼峰命名法,并且不要全部大写,比如将 `enum Status` 改为 `enum QueueStatus`。
3. 错误处理
在函数调用出错时,建议输出错误提示信息,方便调试和排查错误。比如在 `InitQueue` 函数中,如果分配空间失败,可以输出 "allocate memory failed"。
4. 函数参数
建议将函数中不需要修改的参数加上 const 修饰符,以增强代码的可读性和可维护性。
下面是优化后的代码:
```c++
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 6 //最大长度
typedef int QElemType;
typedef struct {
QElemType *data; //初始化的动态分配存储空间
int head; //队头
int tail; //队尾
} SqQueue;
enum QueueStatus {
kError,
kOK
};
//循环队列初始化
QueueStatus InitQueue(SqQueue &Q) {
Q.data = new QElemType[MAXSIZE];
if (!Q.data) {
printf("allocate memory failed\n");
return kError;
}
Q.head = Q.tail = 0; //队空
return kOK;
}
//入队
QueueStatus EnQueue(SqQueue &Q, QElemType e) {
int next = (Q.tail + 1) % MAXSIZE;
//如果队满
if (next == Q.head) {
return kError;
}
Q.data[Q.tail] = e;
Q.tail = next;
return kOK;
}
//出队
QueueStatus DeQueue(SqQueue &Q, QElemType &e) {
//如果队空
if (Q.head == Q.tail) {
return kError;
}
e = Q.data[Q.head];
Q.head = (Q.head + 1) % MAXSIZE;
return kOK;
}
//循环队列长度
int QueueLength(const SqQueue &Q) {
return (Q.tail - Q.head + MAXSIZE) % MAXSIZE;
}
int main() {
QElemType e;
SqQueue Q;
InitQueue(Q);
printf("开始入队\n");
for (int i = 0; i < MAXSIZE - 1; i++) {
scanf("%d", &e);
EnQueue(Q, e);
}
printf("出一个队列元素:\n");
DeQueue(Q, e);
printf("%d\n", e);
printf("再入一个元素\n");
scanf("%d", &e);
EnQueue(Q, e);
printf("全部出队列\n");
for (int i = 0; i < MAXSIZE - 1; i++) {
DeQueue(Q, e);
printf("%d ", e);
}
printf("此时循环队列长度为:%d\n", MAXSIZE - 1 - QueueLength(Q));
return 0;
}
```
此时代码更加规范、清晰易读,也更加易于维护。
相关推荐
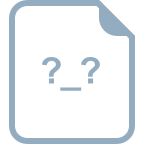
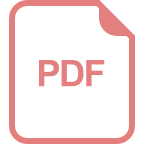
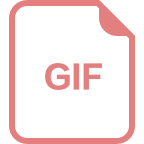















