补全#include<stdio.h> #include<malloc.h> #define MaxSize 50 typedef int ElemType; typedef struct { Elem
时间: 2024-03-22 14:36:28 浏览: 125
#include<stdio.h>
#include<malloc.h>
#define MaxSize 50
typedef int ElemType;
typedef struct
{
ElemType data[MaxSize];
int length;
}SqList;
这段代码是一个C语言的头文件补全,包含了stdio.h和malloc.h两个头文件,并定义了一个常量MaxSize为50。同时,定义了一个类型别名ElemType为int,并声明了一个结构体SqList,其中包含了一个数组data和一个整型变量length。
相关问题
改进以下代码#include<stdio.h> #include<stdlib.h> #include<malloc.h> #define ar arr[]={12,21,2,11,10,8} #define ELEM_TYPE int int ar; //顺序表的创建: typedef struct Sqlist { ELEM_TYPE *data; int length; int SIZE; }Sqlist,*PSqlist; //顺序表的初始化: void Init_Sqlist(P
#include <stdio.h>
#include <stdlib.h>
#include <malloc.h>
#define MAXSIZE 100 // 定义最大长度
typedef int ElemType; // 定义元素类型
typedef struct {
ElemType *data; // 动态分配数组
int length; // 当前长度
int maxSize; // 最大长度
} SqList;
// 初始化顺序表
void InitList(SqList *L) {
L->data = (ElemType *) malloc(sizeof(ElemType) * MAXSIZE); // 动态分配数组
L->length = 0; // 初始长度为0
L->maxSize = MAXSIZE; // 最大长度为MAXSIZE
}
// 插入元素
int Insert(SqList *L, int i, ElemType e) {
// i的合法范围为 1 <= i <= L->length + 1
if (i < 1 || i > L->length + 1) {
return 0; // 插入位置不合法
}
if (L->length >= MAXSIZE) {
return 0; // 当前存储空间已满,不能插入新元素
}
for (int j = L->length; j >= i; j--) {
L->data[j] = L->data[j - 1]; // 将第i个元素及之后的元素后移
}
L->data[i - 1] = e; // 插入新元素
L->length++; // 长度加1
return 1;
}
// 删除元素
int Delete(SqList *L, int i) {
// i的合法范围为 1 <= i <= L->length
if (i < 1 || i > L->length) {
return 0; // 删除位置不合法
}
for (int j = i; j < L->length; j++) {
L->data[j - 1] = L->data[j]; // 将第i+1个元素及之后的元素前移
}
L->length--; // 长度减1
return 1;
}
// 输出顺序表
void PrintList(SqList L) {
for (int i = 0; i < L.length; i++) {
printf("%d ", L.data[i]);
}
printf("\n");
}
int main() {
SqList L;
InitList(&L);
Insert(&L, 1, 1);
Insert(&L, 2, 3);
Insert(&L, 3, 5);
PrintList(L);
Delete(&L, 2);
PrintList(L);
return 0;
}
#include <stdio.h> #include <malloc.h> #define MaxSize 100 typedef char ElemType; typedef struct { ElemType elem[MaxSize]; int top; //栈指针 } SqStack; void InitStack(SqStack **s) //初始化栈s { *s=(SqStack *)malloc(sizeof(SqStack)); (*s)->top= -1 ; } void ClearStack(SqStack *s) //释放栈s { } int StackLength(SqStack *s) //求栈s长度 { int len=0; while(s->top!=-1) { len++; s->top--; } s->top+=len; return len; } int StackEmpty(SqStack *s) //判断栈s是否为空栈 { if( ) return 1; else return 0; } int Push(SqStack *s,ElemType e) //进栈元素e { if(s->top==MaxSize-1) return 0; s->elem[s->top]=e; return 1; } int Pop(SqStack *s,ElemType &e) //出栈一个元素 { if(s->top==-1) return 0; else { s->top--; } return 1; } int GetTop(SqStack *s,ElemType *e) //取栈顶元素 { if(s->top==-1) return 0; else { *e=s->elem[s->top]; } return 1; } void DispStack(SqStack *s) //从栈顶到栈底输出元素 { while(s->top!=-1) { printf("%c\n", ); s->top--; } }
这是一段 C 语言代码,实现了栈的一些基本操作,包括初始化栈、释放栈、求栈的长度、判断栈是否为空、进栈、出栈、取栈顶元素和输出栈中的所有元素。其中:
- `InitStack` 函数用于初始化栈,即为栈分配内存空间并将栈指针指向栈底。
- `ClearStack` 函数用于释放栈,即为栈所占用的内存空间释放掉。
- `StackLength` 函数用于求栈的长度,即栈中元素的个数。
- `StackEmpty` 函数用于判断栈是否为空栈,当栈顶指针为 -1 时,栈为空栈。
- `Push` 函数用于将元素进栈,即将元素插入到栈顶位置。
- `Pop` 函数用于将栈顶元素出栈,即将栈顶元素弹出并删除。
- `GetTop` 函数用于取栈顶元素,即返回栈顶元素的值。
- `DispStack` 函数用于从栈顶到栈底输出栈中的所有元素。
需要注意的是,代码中有些函数的具体实现需要根据具体需求来进行修改,比如 `ClearStack` 函数中需要释放栈中所有元素占用的内存空间。
阅读全文
相关推荐
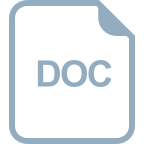
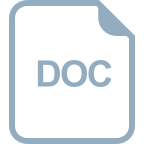
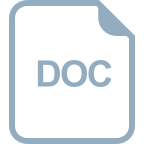



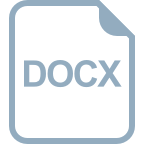
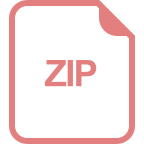
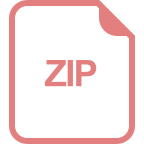
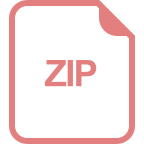