在stm32f407中利用串口1传输结构体数据
时间: 2023-04-05 15:02:42 浏览: 146
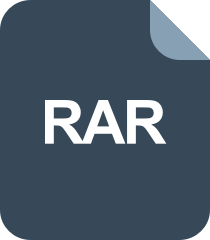
通过USART1 收发数据d.rar_STM32 双串口_stm32 串口 透传_stm32 透传_stm32双串口_亲测可用
可以使用串口发送和接收结构体数据,具体步骤如下:
1. 定义一个结构体,例如:
typedef struct {
uint8_t id;
uint16_t value;
} MyStruct;
2. 在发送端,将结构体转换为字节数组,并通过串口发送:
MyStruct data = {1, 100};
uint8_t buffer[sizeof(MyStruct)];
memcpy(buffer, &data, sizeof(MyStruct));
HAL_UART_Transmit(&huart1, buffer, sizeof(MyStruct), 1000);
3. 在接收端,接收字节数组,并将其转换为结构体:
MyStruct data;
uint8_t buffer[sizeof(MyStruct)];
HAL_UART_Receive(&huart1, buffer, sizeof(MyStruct), 1000);
memcpy(&data, buffer, sizeof(MyStruct));
注意:在发送和接收时,需要保证发送和接收的字节数组大小相同,并且需要使用相同的字节序。
阅读全文
相关推荐
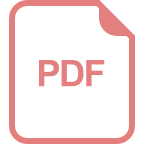
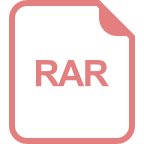

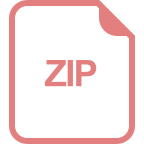

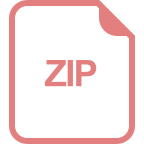
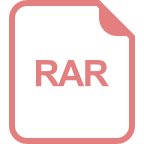
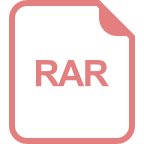
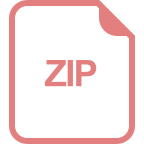
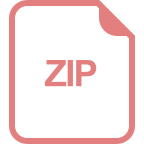
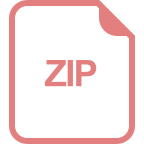
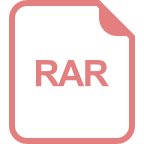
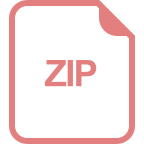
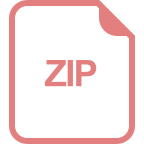
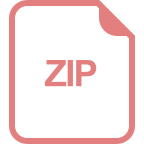
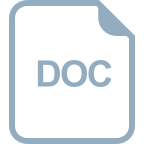

