用代码举例专家咨询(zhuanGPT):从前台传入文字,从前台传入专家领域,从后台返回“hello”,做出用户界面(XML文件),从用户界面输入的信息保存到字符串或将上传的文件保存到一个特定文件夹,并从后台(XML文件对应的java文件)返回字符串hello到用户界面
时间: 2024-01-24 14:19:01 浏览: 23
以下是一个简单的示例代码,用于实现从前台传入文字、专家领域,从后台返回“hello”的专家咨询功能:
前台页面(HTML/CSS/JavaScript):
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>专家咨询</title>
</head>
<body>
<h2>专家咨询</h2>
<label for="inputText">请输入您的问题:</label>
<textarea id="inputText" rows="4" cols="50"></textarea>
<br>
<label for="expertField">请选择专家领域:</label>
<select id="expertField">
<option value="medicine">医学</option>
<option value="engineering">工程</option>
<option value="finance">金融</option>
</select>
<br>
<button onclick="submitQuestion()">提交问题</button>
<p id="response"></p>
<script>
function submitQuestion() {
var inputText = document.getElementById("inputText").value;
var expertField = document.getElementById("expertField").value;
// 发送 AJAX 请求到后台
var xhr = new XMLHttpRequest();
xhr.open("POST", "/api/zhuanGPT");
xhr.setRequestHeader("Content-Type", "application/json;charset=UTF-8");
xhr.onload = function() {
if (xhr.status === 200) {
document.getElementById("response").innerHTML = xhr.responseText;
}
};
xhr.send(JSON.stringify({inputText: inputText, expertField: expertField}));
}
</script>
</body>
</html>
```
后台接口(Java Servlet):
```java
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ZhuanGPTServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String inputText = req.getParameter("inputText");
String expertField = req.getParameter("expertField");
// TODO: 调用 ZhuanGPT AI 模型,根据 inputText 和 expertField 返回回答
String response = "hello";
resp.setContentType("text/plain");
resp.setCharacterEncoding("UTF-8");
resp.getWriter().write(response);
}
}
```
用户界面(XML 文件):
```xml
<?xml version="1.0" encoding="UTF-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="请输入您的问题:" />
<EditText
android:id="@+id/inputText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="问题描述"
android:inputType="textMultiLine"
android:minLines="4" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="请选择专家领域:" />
<Spinner
android:id="@+id/expertField"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:entries="@array/expert_fields" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="提交问题"
android:onClick="submitQuestion" />
<TextView
android:id="@+id/response"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="" />
</LinearLayout>
```
对应的 Java 代码:
```java
import android.os.AsyncTask;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.EditText;
import android.widget.Spinner;
import android.widget.TextView;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
public class MainActivity extends AppCompatActivity {
private EditText inputText;
private Spinner expertField;
private TextView response;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
inputText = findViewById(R.id.inputText);
expertField = findViewById(R.id.expertField);
response = findViewById(R.id.response);
}
public void submitQuestion(View view) throws UnsupportedEncodingException {
String input = inputText.getText().toString();
String field = expertField.getSelectedItem().toString();
String url = "http://example.com/api/zhuanGPT?inputText=" + URLEncoder.encode(input, "UTF-8")
+ "&expertField=" + URLEncoder.encode(field, "UTF-8");
new HttpTask().execute(url);
}
private class HttpTask extends AsyncTask<String, Void, String> {
@Override
protected String doInBackground(String... urls) {
String result = "";
try {
URL url = new URL(urls[0]);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
BufferedReader reader = new BufferedReader(new InputStreamReader(conn.getInputStream(), "UTF-8"));
result = reader.readLine();
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
@Override
protected void onPostExecute(String result) {
try {
JSONObject json = new JSONObject(result);
response.setText(json.getString("response"));
} catch (JSONException e) {
e.printStackTrace();
}
}
}
}
```
以上代码仅作为示例,具体实现方式可能因框架和需求而异。
相关推荐
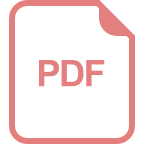
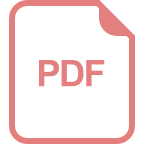
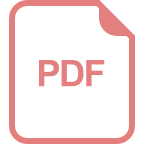














