p ython凯撒加密解密
时间: 2023-07-28 16:07:39 浏览: 136
这里提供一个简单的Python实现凯撒加密和解密的代码:
```python
def caesar_encrypt(plaintext, shift):
"""
凯撒加密函数
:param plaintext: 明文
:param shift: 密钥
:return: 密文
"""
ciphertext = ""
for char in plaintext:
if char.isalpha():
# 将字符转换为 ASCII 码
char_code = ord(char)
# 判断字符是大写字母还是小写字母
if char.isupper():
# 对大写字母进行加密
char_code = (char_code - 65 + shift) % 26 + 65
else:
# 对小写字母进行加密
char_code = (char_code - 97 + shift) % 26 + 97
# 将 ASCII 码转换回字符
char = chr(char_code)
ciphertext += char
return ciphertext
def caesar_decrypt(ciphertext, shift):
"""
凯撒解密函数
:param ciphertext: 密文
:param shift: 密钥
:return: 明文
"""
plaintext = ""
for char in ciphertext:
if char.isalpha():
# 将字符转换为 ASCII 码
char_code = ord(char)
# 判断字符是大写字母还是小写字母
if char.isupper():
# 对大写字母进行解密
char_code = (char_code - 65 - shift) % 26 + 65
else:
# 对小写字母进行解密
char_code = (char_code - 97 - shift) % 26 + 97
# 将 ASCII 码转换回字符
char = chr(char_code)
plaintext += char
return plaintext
```
使用方法:
```python
plaintext = "Hello World!"
shift = 3
ciphertext = caesar_encrypt(plaintext, shift)
print("加密后的密文:", ciphertext)
decrypted_text = caesar_decrypt(ciphertext, shift)
print("解密后的明文:", decrypted_text)
```
输出结果:
```
加密后的密文: Khoor Zruog!
解密后的明文: Hello World!
```
其中,`plaintext` 表示明文字符串,`shift` 表示密钥,`ciphertext` 表示加密后的密文字符串,`decrypted_text` 表示解密后的明文字符串。
阅读全文
相关推荐
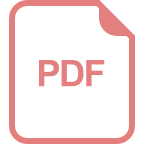
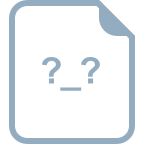





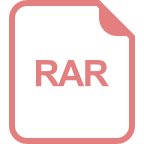
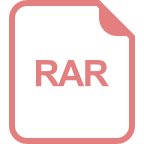
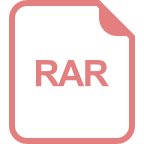
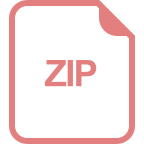
