写一段java代码的飞机大战游戏
时间: 2023-06-05 20:07:43 浏览: 78
很高兴为您提供Java代码的飞机大战游戏。
首先,您需要创建一个名为“PlaneWar”的Java项目,并在其中创建一个名为“GameFrame”的Java类,用于设置游戏界面。代码如下:
```
import javax.swing.*;
import java.awt.*;
public class GameFrame extends JFrame {
public static final int WIDTH = 500;
public static final int HEIGHT = 500;
private JPanel gamePanel;
public GameFrame() {
setTitle("Plane War");
setSize(WIDTH, HEIGHT);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setResizable(false);
gamePanel = new JPanel();
gamePanel.setBackground(Color.BLACK);
gamePanel.setPreferredSize(new Dimension(WIDTH, HEIGHT));
add(gamePanel);
setVisible(true);
}
}
```
接下来,您需要创建名为“Plane”的Java类,用于设置飞机的属性和方法。代码如下:
```
import java.awt.*;
public class Plane {
private int x, y;
private int speed;
private Image image;
public Plane(int x, int y, int speed, String imagePath) {
this.x = x;
this.y = y;
this.speed = speed;
this.image = Toolkit.getDefaultToolkit().getImage(imagePath);
}
public void moveUp() {
if (y > 0) {
y -= speed;
}
}
public void moveDown() {
if (y < GameFrame.HEIGHT - image.getHeight(null)) {
y += speed;
}
}
public void moveLeft() {
if (x > 0) {
x -= speed;
}
}
public void moveRight() {
if (x < GameFrame.WIDTH - image.getWidth(null)) {
x += speed;
}
}
public void draw(Graphics g) {
g.drawImage(image, x, y, null);
}
public Rectangle getRectangle() {
return new Rectangle(x, y, image.getWidth(null), image.getHeight(null));
}
}
```
然后,您需要创建名为“Bullet”的Java类,用于设置子弹的属性和方法。代码如下:
```
import java.awt.*;
public class Bullet {
private int x, y;
private int speed;
private Image image;
public Bullet(int x, int y, int speed, String imagePath) {
this.x = x;
this.y = y;
this.speed = speed;
this.image = Toolkit.getDefaultToolkit().getImage(imagePath);
}
public void move() {
y -= speed;
}
public void draw(Graphics g) {
g.drawImage(image, x, y, null);
}
public Rectangle getRectangle() {
return new Rectangle(x, y, image.getWidth(null), image.getHeight(null));
}
}
```
最后,在“GameFrame”类中添加以下代码来完善游戏:
```
import java.awt.*;
import java.util.ArrayList;
public class GameFrame extends JFrame {
...
private Plane myPlane;
private ArrayList<Bullet> bullets;
private Image background;
public GameFrame() {
...
myPlane = new Plane(WIDTH / 2, HEIGHT - 100, 5, "plane.png");
bullets = new ArrayList<>();
background = Toolkit.getDefaultToolkit().getImage("background.png");
addKeyListener(new GameKeyListener());
new Thread(new GameThread()).start();
}
private void drawGamePanel(Graphics g) {
g.drawImage(background, 0, 0, null);
myPlane.draw(g);
for (Bullet bullet : bullets) {
bullet.draw(g);
}
}
private void moveGameElements() {
for (Bullet bullet : bullets) {
bullet.move();
}
}
...
private class GameThread implements Runnable {
@Override
public void run() {
while (true) {
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
moveGameElements();
gamePanel.repaint();
}
}
}
private class GameKeyListener implements KeyListener {
@Override
public void keyTyped(KeyEvent e) {}
@Override
public void keyPressed(KeyEvent e) {
switch (e.getKeyCode()) {
case KeyEvent.VK_UP:
myPlane.moveUp();
break;
case KeyEvent.VK_DOWN:
myPlane.moveDown();
break;
case KeyEvent.VK_LEFT:
myPlane.moveLeft();
break;
case KeyEvent.VK_RIGHT:
myPlane.moveRight();
break;
case KeyEvent.VK_SPACE:
bullets.add(new Bullet(myPlane.getRectangle().x + myPlane.getRectangle().width / 2 - 10, myPlane.getRectangle().y - 30, 10, "bullet.png"));
break;
}
}
@Override
public void keyReleased(KeyEvent e) {}
}
}
```
现在,您可以在“PlaneWar”项目中添加“plane.png”、“bullet.png”和“background.png”等游戏素材,运行“GameFrame”类,即可玩飞机大战游戏了。
相关推荐
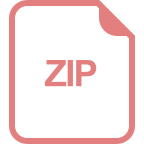














