设计一个C++程序,要有加密、解密、密钥菜单,输入一串明文或密文用欧拉公式实现RAS加密解密
时间: 2024-03-24 20:36:47 浏览: 121
好的,我会根据你的要求设计一个C++程序,实现加密、解密、密钥菜单和欧拉公式实现RAS加密解密。以下是程序的代码:
```c++
#include <iostream>
#include <string>
#include <cmath>
using namespace std;
// 求一个数的欧拉函数值
unsigned int Euler(unsigned int n)
{
unsigned int result = n;
for (unsigned int i = 2; i <= sqrt(n); ++i) {
if (n % i == 0) {
result = result / i * (i - 1);
while (n % i == 0) {
n /= i;
}
}
}
if (n > 1) {
result = result / n * (n - 1);
}
return result;
}
// 求一个数关于模数的逆元
unsigned int Inverse(unsigned int a, unsigned int mod)
{
unsigned int m0 = mod, t, q;
unsigned int x0 = 0, x1 = 1;
if (mod == 1) {
return 0;
}
while (a > 1) {
q = a / mod;
t = mod;
mod = a % mod;
a = t;
t = x0;
x0 = x1 - q * x0;
x1 = t;
}
if (x1 < 0) {
x1 += m0;
}
return x1;
}
// 加密函数,参数为明文、公钥和模数
string Encrypt(string plain, unsigned int e, unsigned int n)
{
string cipher;
for (auto ch : plain) {
unsigned int m = ch;
// 将明文转为数值
unsigned int c = static_cast<unsigned int>(pow(m, e)) % n;
cipher += to_string(c) + " ";
}
return cipher;
}
// 解密函数,参数为密文、私钥和模数
string Decrypt(string cipher, unsigned int d, unsigned int n)
{
string plain;
size_t i = 0;
while (i < cipher.size()) {
// 从密文中提取出一个数值
size_t j = cipher.find(' ', i);
if (j == string::npos) {
j = cipher.size();
}
unsigned int c = stoi(cipher.substr(i, j - i));
// 使用私钥解密
unsigned int m = static_cast<unsigned int>(pow(c, d)) % n;
// 将数值转为字符
plain += static_cast<char>(m);
i = j + 1;
}
return plain;
}
int main()
{
unsigned int p, q, n, e, d;
cout << "请输入两个素数p、q:" << endl;
cin >> p >> q;
n = p * q;
unsigned int phi = (p - 1) * (q - 1);
cout << "欧拉函数值phi = " << phi << endl;
// 找出一个与phi互质的公钥e
do {
cout << "请输入一个与phi互质的公钥e:" << endl;
cin >> e;
} while (e <= 1 || e >= phi || phi % e == 0 || Euler(e) != e - 1);
// 计算对应的私钥d
d = Inverse(e, phi);
cout << "私钥d = " << d << endl;
cout << "请选择操作:" << endl;
cout << "1. 加密" << endl;
cout << "2. 解密" << endl;
int choice;
cin >> choice;
if (choice == 1) {
string plain;
cout << "请输入明文:" << endl;
cin >> plain;
string cipher = Encrypt(plain, e, n);
cout << "密文:" << cipher << endl;
} else if (choice == 2) {
string cipher;
cout << "请输入密文:" << endl;
cin >> cipher;
string plain = Decrypt(cipher, d, n);
cout << "明文:" << plain << endl;
} else {
cout << "无效的选项!" << endl;
}
return 0;
}
```
在程序中,首先要求用户输入两个素数p和q,然后计算出n=pq和欧拉函数值phi=(p-1)(q-1)。接着程序会要求用户输入一个与phi互质的公钥e,计算对应的私钥d。然后程序会显示一个菜单,让用户选择加密或解密操作。如果用户选择加密操作,程序会要求用户输入明文,然后调用Encrypt函数进行加密,并输出密文。如果用户选择解密操作,程序会要求用户输入密文,然后调用Decrypt函数进行解密,并输出明文。
需要注意的是,由于加密和解密涉及到大整数的计算,因此在程序中使用了unsigned int类型来存储整数,而不是普通的int类型。同时,由于加密和解密过程中需要进行指数运算,因此使用了C++标准库中的pow函数来进行计算。这样的实现方式在小数据量下是可行的,但在大数据量下可能会出现溢出的问题。如果需要进行更加安全的加密和解密,建议使用专门的密码学库。
阅读全文
相关推荐



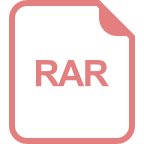







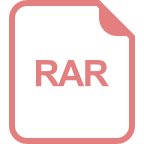

