编写程序,实现队列的基本运算:入队、出队、输出队、取队头、取队尾、求队长、清空队,要求采用顺序循环队实现。
时间: 2023-10-20 09:25:51 浏览: 196
以下是基于Python语言实现的顺序循环队列:
```python
class Queue:
def __init__(self, size):
self.size = size # 队列长度
self.queue = [0] * size # 初始化队列
self.head = 0 # 队首指针
self.tail = 0 # 队尾指针
self.length = 0 # 队列长度
def enqueue(self, value):
"""入队操作"""
if self.length == self.size:
print("队列已满,无法入队")
return False
self.queue[self.tail] = value
self.tail = (self.tail + 1) % self.size
self.length += 1
return True
def dequeue(self):
"""出队操作"""
if self.length == 0:
print("队列为空,无法出队")
return None
value = self.queue[self.head]
self.head = (self.head + 1) % self.size
self.length -= 1
return value
def output(self):
"""输出队列"""
if self.length == 0:
print("队列为空")
return
print("队列元素为:", end="")
i = self.head
while i != self.tail:
print(self.queue[i], end=" ")
i = (i + 1) % self.size
print()
def get_head(self):
"""取队头元素"""
if self.length == 0:
print("队列为空")
return None
return self.queue[self.head]
def get_tail(self):
"""取队尾元素"""
if self.length == 0:
print("队列为空")
return None
return self.queue[(self.tail - 1) % self.size]
def length(self):
"""求队列长度"""
return self.length
def clear(self):
"""清空队列"""
self.head = 0
self.tail = 0
self.length = 0
print("队列已清空")
```
使用示例:
```python
q = Queue(5)
q.enqueue(1)
q.enqueue(2)
q.enqueue(3)
q.output() # 队列元素为:1 2 3
q.dequeue()
q.output() # 队列元素为:2 3
print(q.get_head()) # 2
print(q.get_tail()) # 3
print(q.length) # 2
q.clear()
q.output() # 队列为空
```
阅读全文
相关推荐
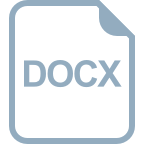


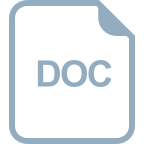
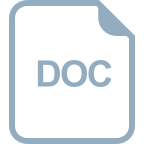
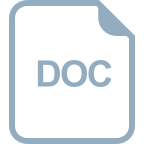
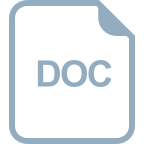
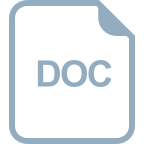
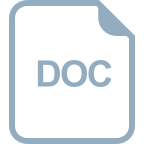
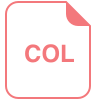






