判断s2是否为s1的子串c语言
时间: 2023-08-21 08:03:33 浏览: 172
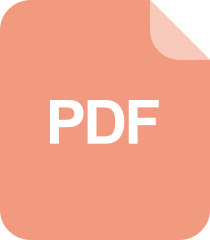
C语言判断字符串是否以str2开头代码
可以使用C语言中的字符串函数strstr()来判断s2是否为s1的子串。该函数的函数原型为char *strstr(const char *haystack, const char *needle),其中haystack表示要查找的字符串,needle表示要查找的子串。如果查找成功,函数返回子串在字符串中第一次出现的地址;如果查找失败,函数返回NULL。
下面是使用strstr()实现判断s2是否为s1的子串的代码示例:
```c
#include <stdio.h>
#include <string.h>
int main() {
char s1[100], s2[100];
printf("请输入s1和s2:");
scanf("%s%s", s1, s2);
if (strstr(s1, s2) != NULL) {
printf("s2是s1的子串\n");
} else {
printf("s2不是s1的子串\n");
}
return 0;
}
```
阅读全文
相关推荐
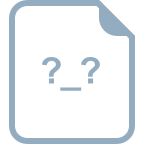













