请用pyside6写一个可以展示大型tif,png类型的界面,可以放大缩小,拖动
时间: 2023-10-02 15:05:14 浏览: 93
好的,以下是一个简单的示例代码:
```python
import sys
from PySide6.QtGui import QImage, QPixmap
from PySide6.QtWidgets import QApplication, QGraphicsScene, QGraphicsView, QMainWindow
class ImageViewer(QMainWindow):
def __init__(self, parent=None):
super().__init__(parent)
self.scene = QGraphicsScene(self)
self.view = QGraphicsView(self.scene)
self.setCentralWidget(self.view)
# 设置初始大小和中心点
self.view.setRenderHints(QPainter.Antialiasing | QPainter.SmoothPixmapTransform)
self.view.setViewportUpdateMode(QGraphicsView.FullViewportUpdate)
self.view.setDragMode(QGraphicsView.ScrollHandDrag)
self.view.setOptimizationFlag(QGraphicsView.DontAdjustForAntialiasing, True)
self.view.setOptimizationFlag(QGraphicsView.DontSavePainterState, True)
self.view.setOptimizationFlag(QGraphicsView.DontAdjustForAntialiasing, True)
self.view.setOptimizationFlag(QGraphicsView.DontAdjustForIndirectPainting, True)
# 打开并显示图像
self.image = QImage("test.tif")
self.pixmap = QPixmap.fromImage(self.image)
self.scene.addPixmap(self.pixmap)
# 调整视图大小以适应场景
self.view.fitInView(self.scene.sceneRect(), Qt.KeepAspectRatio)
def wheelEvent(self, event):
# 缩放图像
if event.angleDelta().y() > 0:
self.view.scale(1.2, 1.2)
else:
self.view.scale(0.8, 0.8)
def keyPressEvent(self, event):
# 按住空格键并拖动视图以移动图像
if event.key() == Qt.Key_Space:
self.view.setDragMode(QGraphicsView.ScrollHandDrag)
else:
super().keyPressEvent(event)
def keyReleaseEvent(self, event):
# 松开空格键以停止拖动视图
if event.key() == Qt.Key_Space:
self.view.setDragMode(QGraphicsView.NoDrag)
else:
super().keyReleaseEvent(event)
if __name__ == "__main__":
app = QApplication(sys.argv)
window = ImageViewer()
window.show()
sys.exit(app.exec())
```
这里使用了 PySide6 的 QGraphicsView 和 QGraphicsScene 类来实现图像的显示和缩放、拖动。在示例中,我们打开了一个名为 "test.tif" 的图像,并将其添加到场景中。我们还覆盖了 wheelEvent()、keyPressEvent() 和 keyReleaseEvent() 方法以允许用户缩放和拖动图像。
阅读全文
相关推荐
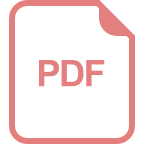















