python opencv读标记文件标记图像
时间: 2023-10-10 09:06:03 浏览: 49
这里提供一个简单的示例代码,使用Python OpenCV读取标记文件和标记图像:
```python
import cv2
# 读取标记文件和标记图像
label_file = "label.txt"
image_file = "image.jpg"
# 读取标记文件
with open(label_file, "r") as f:
lines = f.readlines()
# 解析标记矩形框的坐标和标签
boxes = []
labels = []
for line in lines:
box = [int(x) for x in line.strip().split()[1:]]
label = line.strip().split()[0]
boxes.append(box)
labels.append(label)
# 读取标记图像
image = cv2.imread(image_file)
# 在图像上绘制矩形框和标签
for box, label in zip(boxes, labels):
x1, y1, x2, y2 = box
cv2.rectangle(image, (x1, y1), (x2, y2), (0, 255, 0), 2)
cv2.putText(image, label, (x1, y1-5), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示标记图像
cv2.imshow("labeled image", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`label.txt`文件包含了每个矩形框的标签和坐标信息,格式如下:
```
label1 x1 y1 x2 y2
label2 x1 y1 x2 y2
...
```
`image.jpg`是待标记的图像文件。代码先读取标记文件中的矩形框坐标和标签,然后读取标记图像,最后使用`cv2.rectangle()`和`cv2.putText()`函数在图像上绘制矩形框和标签。最后使用`cv2.imshow()`函数显示标记图像。
相关推荐
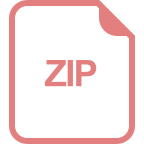
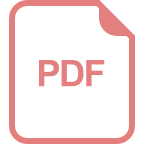
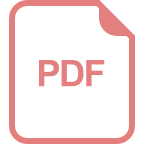














