基于javaweb和数据据写一个简单的图书增删改查代码
时间: 2024-05-15 08:19:09 浏览: 81
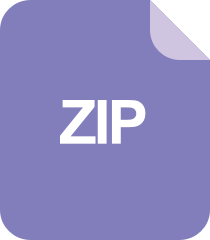
javaweb图书信息增删改查

好的,以下是一个简单的基于JavaWeb和数据库的图书增删改查代码示例,供您参考:
```
// 前端JSP界面中的增删改查操作对应着不同的URL请求
@WebServlet("/book")
public class BookServlet extends HttpServlet {
// 初始化DAO对象
private BookDao bookDao = new BookDao();
// 处理GET请求,即展示所有图书信息
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
List<Book> books = bookDao.getAllBooks(); // 获取所有图书信息
request.setAttribute("books", books); // 设置请求属性
request.getRequestDispatcher("book.jsp").forward(request, response); // 转发JSP界面
}
// 处理POST请求,即操作图书信息
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 获取操作类型
String action = request.getParameter("action");
if (action == null) { // 如果未指定操作类型,则返回错误信息
response.getWriter().println("Invalid Request!");
return;
}
if (action.equals("add")) { // 添加图书信息
String name = request.getParameter("name");
String author = request.getParameter("author");
int price = Integer.parseInt(request.getParameter("price"));
int inventory = Integer.parseInt(request.getParameter("inventory"));
bookDao.addBook(new Book(name, author, price, inventory)); // 调用DAO对象的添加方法
} else if (action.equals("delete")) { // 删除图书信息
int id = Integer.parseInt(request.getParameter("id"));
bookDao.deleteBook(id); // 调用DAO对象的删除方法
} else if (action.equals("update")) { // 更新图书信息
int id = Integer.parseInt(request.getParameter("id"));
String name = request.getParameter("name");
String author = request.getParameter("author");
int price = Integer.parseInt(request.getParameter("price"));
int inventory = Integer.parseInt(request.getParameter("inventory"));
bookDao.updateBook(new Book(id, name, author, price, inventory)); // 调用DAO对象的更新方法
} else { // 如果操作类型无效,则返回错误信息
response.getWriter().println("Invalid Action: " + action);
return;
}
response.sendRedirect("book"); // 重定向GET请求,展示所有图书信息
}
}
```
其中,DAO对象是负责与数据库交互的数据访问层,示例代码如下:
```
public class BookDao {
// 数据库连接信息
private static final String DRIVER = "com.mysql.jdbc.Driver";
private static final String URL = "jdbc:mysql://localhost:3306/library";
private static final String USERNAME = "root";
private static final String PASSWORD = "password";
static { // 在加载DAO类时,自动加载数据库驱动
try {
Class.forName(DRIVER);
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
// 获取所有图书信息
public List<Book> getAllBooks() {
List<Book> books = new ArrayList<>();
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
stmt = conn.createStatement();
rs = stmt.executeQuery("SELECT * FROM book");
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
String author = rs.getString("author");
int price = rs.getInt("price");
int inventory = rs.getInt("inventory");
books.add(new Book(id, name, author, price, inventory));
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
return books;
}
// 根据ID号获取图书信息
public Book getBook(int id) {
Book book = null;
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
pstmt = conn.prepareStatement("SELECT * FROM book WHERE id = ?");
pstmt.setInt(1, id);
rs = pstmt.executeQuery();
if (rs.next()) {
String name = rs.getString("name");
String author = rs.getString("author");
int price = rs.getInt("price");
int inventory = rs.getInt("inventory");
book = new Book(id, name, author, price, inventory);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) {
rs.close();
}
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
return book;
}
// 添加新图书信息
public void addBook(Book book) {
Connection conn = null;
PreparedStatement pstmt = null;
try {
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
pstmt = conn.prepareStatement("INSERT INTO book (name, author, price, inventory) VALUES (?, ?, ?, ?)");
pstmt.setString(1, book.getName());
pstmt.setString(2, book.getAuthor());
pstmt.setInt(3, book.getPrice());
pstmt.setInt(4, book.getInventory());
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// 删除图书信息
public void deleteBook(int id) {
Connection conn = null;
PreparedStatement pstmt = null;
try {
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
pstmt = conn.prepareStatement("DELETE FROM book WHERE id = ?");
pstmt.setInt(1, id);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// 更新图书信息
public void updateBook(Book book) {
Connection conn = null;
PreparedStatement pstmt = null;
try {
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
pstmt = conn.prepareStatement("UPDATE book SET name = ?, author = ?, price = ?, inventory = ? WHERE id = ?");
pstmt.setString(1, book.getName());
pstmt.setString(2, book.getAuthor());
pstmt.setInt(3, book.getPrice());
pstmt.setInt(4, book.getInventory());
pstmt.setInt(5, book.getId());
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
以上就是一个简单的基于JavaWeb和数据库的图书增删改查代码示例,希望对您有所帮助!
阅读全文
相关推荐
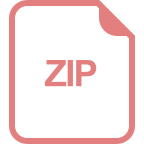
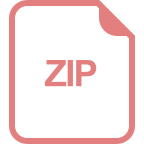
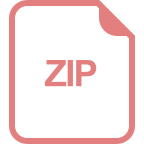
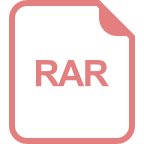
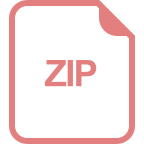

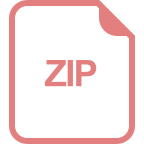
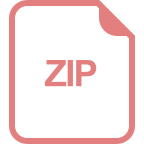
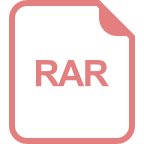
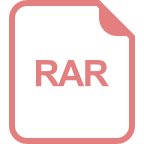
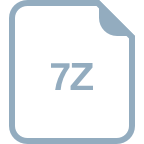
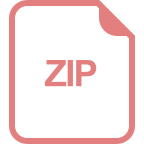
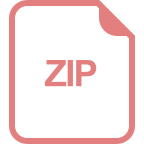
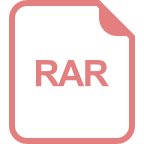
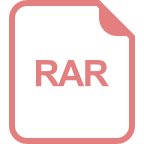
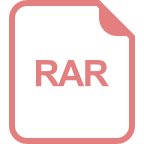