javaweb增删改查代码
时间: 2023-07-07 20:40:58 浏览: 46
这是一个简单的 JavaWeb 增删改查代码示例,主要使用了 JDBC 连接数据库实现:
1. 添加数据:
```java
public void add(Student student) throws SQLException {
Connection conn = getConnection();
PreparedStatement pstmt = conn.prepareStatement("INSERT INTO student(name, age, gender) VALUES(?,?,?)");
pstmt.setString(1, student.getName());
pstmt.setInt(2, student.getAge());
pstmt.setString(3, student.getGender());
pstmt.executeUpdate();
pstmt.close();
conn.close();
}
```
2. 删除数据:
```java
public void delete(int id) throws SQLException {
Connection conn = getConnection();
PreparedStatement pstmt = conn.prepareStatement("DELETE FROM student WHERE id=?");
pstmt.setInt(1, id);
pstmt.executeUpdate();
pstmt.close();
conn.close();
}
```
3. 修改数据:
```java
public void update(Student student) throws SQLException {
Connection conn = getConnection();
PreparedStatement pstmt = conn.prepareStatement("UPDATE student SET name=?, age=?, gender=? WHERE id=?");
pstmt.setString(1, student.getName());
pstmt.setInt(2, student.getAge());
pstmt.setString(3, student.getGender());
pstmt.setInt(4, student.getId());
pstmt.executeUpdate();
pstmt.close();
conn.close();
}
```
4. 查询数据:
```java
public List<Student> query() throws SQLException {
Connection conn = getConnection();
PreparedStatement pstmt = conn.prepareStatement("SELECT * FROM student");
ResultSet rs = pstmt.executeQuery();
List<Student> list = new ArrayList<>();
while(rs.next()) {
Student student = new Student();
student.setId(rs.getInt("id"));
student.setName(rs.getString("name"));
student.setAge(rs.getInt("age"));
student.setGender(rs.getString("gender"));
list.add(student);
}
rs.close();
pstmt.close();
conn.close();
return list;
}
```
注意:以上代码仅供参考,实际使用时需要根据具体情况进行修改。同时需要注意数据库连接的释放和异常处理。
相关推荐
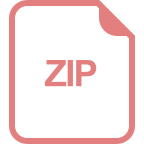














