用C语言编写算法逆置一个带头结点的双链表。
时间: 2024-04-06 13:08:22 浏览: 149
下面是C语言代码实现双向链表逆置的算法:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
struct Node* prev;
};
void insertAtEnd(struct Node** head_ref, int new_data)
{
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
struct Node* last = *head_ref;
new_node->data = new_data;
new_node->next = NULL;
if (*head_ref == NULL) {
new_node->prev = NULL;
*head_ref = new_node;
return;
}
while (last->next != NULL)
last = last->next;
last->next = new_node;
new_node->prev = last;
return;
}
void printList(struct Node* node)
{
struct Node* last;
printf("\nTraversal in forward direction \n");
while (node != NULL) {
printf(" %d ", node->data);
last = node;
node = node->next;
}
printf("\nTraversal in reverse direction \n");
while (last != NULL) {
printf(" %d ", last->data);
last = last->prev;
}
}
void reverse(struct Node** head_ref)
{
struct Node* current = *head_ref;
struct Node* temp = NULL;
while (current != NULL) {
temp = current->prev;
current->prev = current->next;
current->next = temp;
current = current->prev;
}
if (temp != NULL)
*head_ref = temp->prev;
}
int main()
{
struct Node* head = NULL;
insertAtEnd(&head, 1);
insertAtEnd(&head, 2);
insertAtEnd(&head, 3);
insertAtEnd(&head, 4);
insertAtEnd(&head, 5);
printf("Original doubly linked list is: ");
printList(head);
reverse(&head);
printf("\nReversed doubly linked list is: ");
printList(head);
return 0;
}
```
这段代码定义了一个双向链表的结构体 `Node`,并提供了向链表尾部插入节点的函数 `insertAtEnd`,遍历链表的函数 `printList`,以及逆置链表的函数 `reverse`。
在 `main` 函数中,我们创建了一个带头结点的双向链表,并调用 `printList` 函数打印出原始链表的内容。然后我们调用 `reverse` 函数逆置链表,并再次调用 `printList` 函数打印出逆置后的链表的内容。
逆置链表的实现方法是从头结点开始,依次交换前驱和后继指针,直到链表末尾。最后再把头结点指向链表末尾,完成逆置。
阅读全文
相关推荐
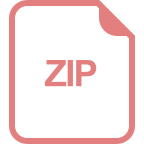
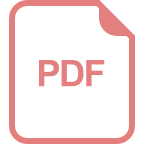
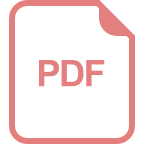














