使用SM4加密文件以后如何解密,请给出基于java的代码示例
时间: 2024-03-05 20:48:39 浏览: 16
以下是基于Java语言的SM4文件加密与解密示例代码:
```java
import java.io.*;
import java.security.*;
import javax.crypto.*;
import javax.crypto.spec.*;
public class SM4Demo {
public static void main(String[] args) throws Exception {
String inputFilePath = "input.txt"; // 待加密的文件路径
String outputFilePath = "output.txt"; // 加密后的文件路径
String decryptedFilePath = "decrypted.txt"; // 解密后的文件路径
String password = "1234567890abcdef"; // 密钥
// 加密文件
encryptFile(inputFilePath, outputFilePath, password);
// 解密文件
decryptFile(outputFilePath, decryptedFilePath, password);
}
/**
* 加密文件
* @param inputFilePath 待加密的文件路径
* @param outputFilePath 加密后的文件路径
* @param password 密钥
*/
public static void encryptFile(String inputFilePath, String outputFilePath, String password) throws Exception {
// 生成密钥
byte[] keyBytes = password.getBytes("UTF-8");
SecretKeySpec keySpec = new SecretKeySpec(keyBytes, "SM4");
// 初始化加密器
Cipher cipher = Cipher.getInstance("SM4/ECB/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, keySpec);
// 打开输入文件和输出文件
FileInputStream in = new FileInputStream(inputFilePath);
FileOutputStream out = new FileOutputStream(outputFilePath);
// 加密文件
byte[] buffer = new byte[4096];
int len;
while ((len = in.read(buffer)) > 0) {
byte[] cipherData = cipher.update(buffer, 0, len);
if (cipherData != null) {
out.write(cipherData);
}
}
byte[] cipherData = cipher.doFinal();
if (cipherData != null) {
out.write(cipherData);
}
// 关闭输入文件和输出文件
in.close();
out.close();
}
/**
* 解密文件
* @param inputFilePath 待解密的文件路径
* @param outputFilePath 解密后的文件路径
* @param password 密钥
*/
public static void decryptFile(String inputFilePath, String outputFilePath, String password) throws Exception {
// 生成密钥
byte[] keyBytes = password.getBytes("UTF-8");
SecretKeySpec keySpec = new SecretKeySpec(keyBytes, "SM4");
// 初始化解密器
Cipher cipher = Cipher.getInstance("SM4/ECB/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, keySpec);
// 打开输入文件和输出文件
FileInputStream in = new FileInputStream(inputFilePath);
FileOutputStream out = new FileOutputStream(outputFilePath);
// 解密文件
byte[] buffer = new byte[4096];
int len;
while ((len = in.read(buffer)) > 0) {
byte[] plainData = cipher.update(buffer, 0, len);
if (plainData != null) {
out.write(plainData);
}
}
byte[] plainData = cipher.doFinal();
if (plainData != null) {
out.write(plainData);
}
// 关闭输入文件和输出文件
in.close();
out.close();
}
}
```
以上代码使用了Java标准库中的`javax.crypto`包来完成SM4加密和解密操作。在加密和解密时,需要指定密钥和加密模式,这里使用了ECB模式和PKCS5Padding填充方式。需要注意的是,这里使用了固定长度的密钥,实际应用中应该使用更加安全的密钥生成方式。
相关推荐
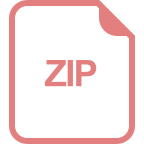
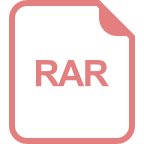
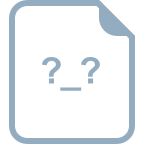














