n个不同整数中取k个数字,输出显示所有的组合
时间: 2023-08-09 20:05:09 浏览: 54
以下是一个Python实现的例子:
```python
import itertools
n = 5
k = 3
# 生成n个不同的整数
nums = list(range(1, n+1))
# 获取所有长度为k的组合
comb = itertools.combinations(nums, k)
# 输出所有组合
for c in comb:
print(c)
```
输出结果为:
```
(1, 2, 3)
(1, 2, 4)
(1, 2, 5)
(1, 3, 4)
(1, 3, 5)
(1, 4, 5)
(2, 3, 4)
(2, 3, 5)
(2, 4, 5)
(3, 4, 5)
```
这里使用了Python标准库中的`itertools.combinations`函数来获取所有长度为k的组合。
相关问题
n个不同整数中取k个数字,输出显示所有的组合,用c++写一下
好的,以下是用C++实现的代码:
```cpp
#include <iostream>
#include <vector>
using namespace std;
void generate_combinations(int n, int k, vector<int>& nums, vector<vector<int>>& res, vector<int>& curr, int start) {
if (curr.size() == k) {
res.push_back(curr);
return;
}
for (int i = start; i <= n; ++i) {
curr.push_back(nums[i-1]);
generate_combinations(n, k, nums, res, curr, i+1);
curr.pop_back();
}
}
int main() {
int n = 5;
int k = 3;
// 生成n个不同的整数
vector<int> nums(n);
for (int i = 0; i < n; ++i) {
nums[i] = i+1;
}
// 获取所有长度为k的组合
vector<vector<int>> res;
vector<int> curr;
generate_combinations(n, k, nums, res, curr, 1);
// 输出所有组合
for (const auto& v : res) {
for (int num : v) {
cout << num << " ";
}
cout << endl;
}
return 0;
}
```
这里使用了递归的方式来生成所有的组合,代码中`generate_combinations`函数的参数含义如下:
- `n`:总共有多少个数字;
- `k`:每个组合中包含多少个数字;
- `nums`:存储所有数字的vector,每个数字都不相同;
- `res`:存储所有组合的vector<vector<int>>,每个组合是一个vector<int>;
- `curr`:当前正在生成的组合;
- `start`:从哪个数字开始生成。
在函数中,如果当前正在生成的组合的长度等于k,就将它添加到res中;否则,从start开始,依次将nums中的数字添加到curr中,并递归生成下一个数字。
输入正整数n和k,要求输出由k个1-n组成的组合
抱歉,我可以回答此问题。这是一个组合问题,可以使用递归或迭代方法来实现。以下是一份Python代码示例:
```python
def combinations(n, k):
if k <= 0:
return [[]]
if n < k:
return []
if n == k:
return [[i for i in range(1, n+1)]]
combs = combinations(n-1, k)
combs.extend([c+[n] for c in combinations(n-1, k-1)])
return combs
n = int(input("请输入正整数n:"))
k = int(input("请输入正整数k:"))
result = combinations(n, k)
print(f"{n}个数字中选取{k}个数字的组合有{len(result)}种:")
for r in result:
print(r)
```
输入示例:
```text
请输入正整数n:5
请输入正整数k:3
```
输出示例:
```text
5个数字中选取3个数字的组合有10种:
[1, 2, 3]
[1, 2, 4]
[1, 2, 5]
[1, 3, 4]
[1, 3, 5]
[1, 4, 5]
[2, 3, 4]
[2, 3, 5]
[2, 4, 5]
[3, 4, 5]
```
相关推荐
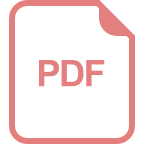
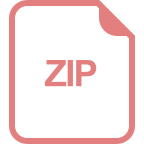
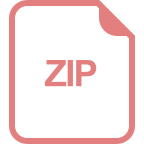












