帮我写一个人脸验证系统的Python代码
时间: 2023-08-28 20:25:55 浏览: 34
好的,以下是一个使用Python编写的简单人脸验证系统的示例代码。请注意,这只是一个基本的示例,并不是完整的系统。
```python
import cv2
import numpy as np
import face_recognition
# 采集面部特征信息
def capture_face():
# 打开摄像头
cap = cv2.VideoCapture(0)
while True:
# 读取每一帧图像
ret, frame = cap.read()
# 将图像调整为1/4大小以加快处理速度
small_frame = cv2.resize(frame, (0, 0), fx=0.25, fy=0.25)
# 转换图像颜色空间
rgb_small_frame = small_frame[:, :, ::-1]
# 检测面部特征信息
face_locations = face_recognition.face_locations(rgb_small_frame)
# 如果检测到面部特征信息,则返回
if len(face_locations) > 0:
# 获取面部特征信息的位置
top, right, bottom, left = face_locations[0]
# 调整面部特征信息的位置
top *= 4
right *= 4
bottom *= 4
left *= 4
# 保存面部特征信息的位置和图像
face_image = frame[top:bottom, left:right]
return face_image
# 如果未检测到面部特征信息,则继续读取下一帧图像
cv2.imshow('Video', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放摄像头并关闭窗口
cap.release()
cv2.destroyAllWindows()
# 存储面部特征信息
def store_face():
# 采集面部特征信息
face_image = capture_face()
# 提取面部特征信息
face_encoding = face_recognition.face_encodings(face_image)[0]
# 将面部特征信息保存到文件中
np.savetxt('face_encodings.txt', face_encoding)
# 验证身份
def verify_face():
# 读取面部特征信息
face_encoding = np.loadtxt('face_encodings.txt')
# 采集面部特征信息
face_image = capture_face()
# 提取面部特征信息
face_encoding_test = face_recognition.face_encodings(face_image)[0]
# 比对面部特征信息
results = face_recognition.compare_faces([face_encoding], face_encoding_test)
# 如果匹配,则打印验证通过的信息
if results[0]:
print('Verification passed')
# 如果不匹配,则打印验证失败的信息
else:
print('Verification failed')
# 主函数
if __name__ == '__main__':
# 存储面部特征信息
store_face()
# 验证身份
verify_face()
```
该代码使用了开源的face_recognition库来进行面部特征信息的采集和比对。在运行代码之前,需要先安装face_recognition库和OpenCV库。
相关推荐
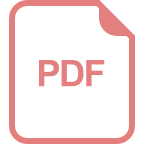
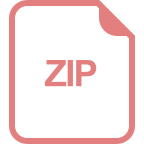
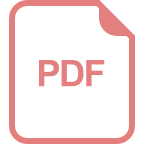














