加载3D模型的3D代码
时间: 2024-05-16 15:15:42 浏览: 11
加载3D模型的3D代码通常使用OpenGL或WebGL等图形库来实现。以下是一个使用WebGL加载3D模型的示例代码:
```javascript
// 获取WebGL上下文
const gl = canvas.getContext('webgl');
// 定义顶点着色器
const vertexShaderSource = `
attribute vec4 a_Position;
attribute vec4 a_Color;
attribute vec3 a_Normal;
uniform mat4 u_ModelMatrix;
uniform mat4 u_ViewMatrix;
uniform mat4 u_ProjectionMatrix;
varying vec4 v_Color;
varying vec3 v_Normal;
void main() {
gl_Position = u_ProjectionMatrix * u_ViewMatrix * u_ModelMatrix * a_Position;
v_Color = a_Color;
v_Normal = mat3(u_ModelMatrix) * a_Normal;
}
`;
// 定义片元着色器
const fragmentShaderSource = `
precision mediump float;
uniform vec3 u_LightDirection;
varying vec4 v_Color;
varying vec3 v_Normal;
void main() {
vec3 normal = normalize(v_Normal);
float intensity = dot(normal, -u_LightDirection);
gl_FragColor = vec4(v_Color.rgb * intensity, v_Color.a);
}
`;
// 创建顶点着色器
const vertexShader = gl.createShader(gl.VERTEX_SHADER);
gl.shaderSource(vertexShader, vertexShaderSource);
gl.compileShader(vertexShader);
// 创建片元着色器
const fragmentShader = gl.createShader(gl.FRAGMENT_SHADER);
gl.shaderSource(fragmentShader, fragmentShaderSource);
gl.compileShader(fragmentShader);
// 创建着色器程序
const program = gl.createProgram();
gl.attachShader(program, vertexShader);
gl.attachShader(program, fragmentShader);
gl.linkProgram(program);
// 获取顶点属性和uniform变量的位置
const a_Position = gl.getAttribLocation(program, 'a_Position');
const a_Color = gl.getAttribLocation(program, 'a_Color');
const a_Normal = gl.getAttribLocation(program, 'a_Normal');
const u_ModelMatrix = gl.getUniformLocation(program, 'u_ModelMatrix');
const u_ViewMatrix = gl.getUniformLocation(program, 'u_ViewMatrix');
const u_ProjectionMatrix = gl.getUniformLocation(program, 'u_ProjectionMatrix');
const u_LightDirection = gl.getUniformLocation(program, 'u_LightDirection');
// 加载3D模型数据
const positions = [/* 顶点坐标数据 */];
const colors = [/* 顶点颜色数据 */];
const normals = [/* 顶点法向量数据 */];
const indices = [/* 三角形索引数据 */];
// 创建顶点缓冲器
const positionBuffer = gl.createBuffer();
gl.bindBuffer(gl.ARRAY_BUFFER, positionBuffer);
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(positions), gl.STATIC_DRAW);
gl.vertexAttribPointer(a_Position, 3, gl.FLOAT, false, 0, 0);
gl.enableVertexAttribArray(a_Position);
const colorBuffer = gl.createBuffer();
gl.bindBuffer(gl.ARRAY_BUFFER, colorBuffer);
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(colors), gl.STATIC_DRAW);
gl.vertexAttribPointer(a_Color, 4, gl.FLOAT, false, 0, 0);
gl.enableVertexAttribArray(a_Color);
const normalBuffer = gl.createBuffer();
gl.bindBuffer(gl.ARRAY_BUFFER, normalBuffer);
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(normals), gl.STATIC_DRAW);
gl.vertexAttribPointer(a_Normal, 3, gl.FLOAT, false, 0, 0);
gl.enableVertexAttribArray(a_Normal);
const indexBuffer = gl.createBuffer();
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, indexBuffer);
gl.bufferData(gl.ELEMENT_ARRAY_BUFFER, new Uint16Array(indices), gl.STATIC_DRAW);
// 绘制3D模型
gl.useProgram(program);
gl.uniformMatrix4fv(u_ModelMatrix, false, new Float32Array(/* 模型矩阵数据 */));
gl.uniformMatrix4fv(u_ViewMatrix, false, new Float32Array(/* 视图矩阵数据 */));
gl.uniformMatrix4fv(u_ProjectionMatrix, false, new Float32Array(/* 投影矩阵数据 */));
gl.uniform3fv(u_LightDirection, new Float32Array(/* 光源方向数据 */));
gl.drawElements(gl.TRIANGLES, indices.length, gl.UNSIGNED_SHORT, 0);
```
相关推荐
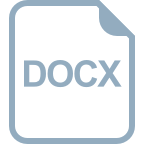














