字符串每个单词首字母大写
时间: 2023-07-25 16:08:36 浏览: 116
可以使用 Python 的 title() 方法来实现字符串每个单词首字母大写的操作,示例如下:
```python
s = "hello world"
s = s.title()
print(s)
```
输出结果为:
```
Hello World
```
title() 方法会将字符串中每个单词的首字母转换为大写,并返回转换后的字符串。注意,该方法不会改变原始字符串,而是返回一个新的字符串。
相关问题
python中将字符串每个单词首字母大写,不用title 和capitalize
在Python中,如果你想将字符串中每个单词的首字母转换成大写,但又不想使用`title()`或`capitalize()`方法,可以使用切片和字符串连接的方式来实现。这里有一个简单的示例:
```python
def capitalize_each_word(s):
words = s.split() # 将字符串分割成单词列表
capitalized_words = [word[0].upper() + word[1:] for word in words] # 首字母大写,其余部分不变
return ' '.join(capitalized_words) # 再将单词列表合并回字符串
# 使用示例
input_str = "hello world, how are you?"
output_str = capitalize_each_word(input_str)
print(output_str) # 输出: Hello World, How Are You?
```
这个函数通过列表推导式完成了单词首字母大写的操作,然后使用`join()`方法将它们重新组合成一个字符串。
如何在C语言中使用for循环实现字符串每个单词首字母大写?
在C语言中,为了将字符串中的每个单词首字母转换为大写,你可以使用`strtok()`函数分隔单词,然后对每个单词应用`toupper()`函数。这里是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
void capitalize_first_char(char *str) {
char *token;
// 分割字符串并获取单词
token = strtok(str, " "); // 使用空格作为分隔符
while (token != NULL) {
if (*token) { // 避免处理空单词
// 将第一个字符转为大写
*token = toupper(*token);
// 把当前单词的剩余部分连接回原字符串
strcat(str, token + 1);
// 继续分割下一个单词
str = token + 1; // 移动到下一个单词开始的位置
}
// 获取下一个单词
token = strtok(NULL, " ");
}
}
int main() {
char input[] = "hello world this is a test";
capitalize_first_char(input);
printf("%s\n", input); // 输出: Hello World This Is A Test
return 0;
}
阅读全文
相关推荐
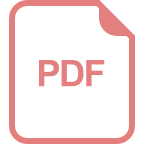
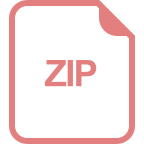
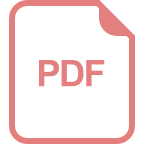


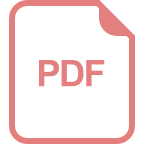










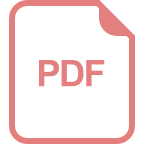