给一个字符串heLLo woRld,并设置种模式,模 式1将字符串首字母大写,其他小写;模式2将字符 串中所有字母大写;模式3将字符串每个单词的首 字母大写,其他字母小写。键盘选择模式,然后输 出结果C语言
时间: 2024-12-25 18:29:11 浏览: 8
在C语言中,你可以创建一个函数来接受字符串和模式作为参数,然后根据模式来转换字符串。以下是三种模式的实现:
1. 模式1(仅首字母大写):
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
void convert_case_1(char *str) {
int length = strlen(str);
if (length > 0)
str[0] = toupper(str[0]); // 首字母大写
}
int main() {
char input[] = "heLLo woRld";
int mode = 1; // 设定模式
switch (mode) {
case 1:
convert_case_1(input);
break;
// 其他模式可以添加...
}
printf("Mode 1 result: %s\n", input);
return 0;
}
```
2. 模式2(全大写):
```c
void convert_case_2(char *str) {
int length = strlen(str);
for (int i = 0; i < length; i++) {
str[i] = toupper(str[i]); // 所有字母转大写
}
}
// 主函数同上,只需替换convert_case_1为convert_case_2
```
3. 模式3(单词首字母大写):
```c
void convert_case_3(char *str) {
for (char *word = strtok(str, " "); word != NULL; word = strtok(NULL, " ")) {
if (word[0]) { // 非空单词
word[0] = toupper(word[0]); // 首字母大写
for (int i = 1; word[i]; i++) {
word[i] = tolower(word[i]); // 其他字母小写
}
}
}
}
// 主函数同上,只需替换convert_case_1为convert_case_3
```
在主函数中,你可以增加一个循环让用户输入模式,然后根据用户的选择调用相应的函数。
阅读全文
相关推荐
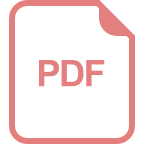
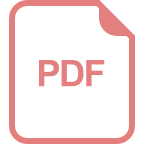
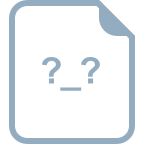















