用C语言写一段代码,要求是链表的初始化、插入、删除、打印、销毁
时间: 2023-09-28 13:11:16 浏览: 95
下面是一个简单的链表操作的 C 语言代码,包括链表的初始化、插入、删除、打印和销毁函数:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node *next;
} Node;
// 初始化链表
Node *initList() {
Node *head = (Node*) malloc(sizeof(Node));
head->next = NULL;
return head;
}
// 在链表末尾插入节点
void insert(Node *head, int val) {
Node *p = head;
while (p->next != NULL) {
p = p->next;
}
Node *newNode = (Node*) malloc(sizeof(Node));
newNode->data = val;
newNode->next = NULL;
p->next = newNode;
}
// 删除链表中第一个值为 val 的节点
void delete(Node *head, int val) {
Node *p = head->next;
Node *pre = head;
while (p != NULL && p->data != val) {
pre = p;
p = p->next;
}
if (p != NULL) {
pre->next = p->next;
free(p);
}
}
// 打印链表中所有节点的值
void printList(Node *head) {
Node *p = head->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
// 销毁链表
void destroyList(Node *head) {
Node *p = head->next;
while (p != NULL) {
Node *tmp = p;
p = p->next;
free(tmp);
}
free(head);
}
int main() {
Node *head = initList();
// 插入节点
insert(head, 1);
insert(head, 2);
insert(head, 3);
// 打印链表
printList(head);
// 删除节点
delete(head, 2);
// 再次打印链表
printList(head);
// 销毁链表
destroyList(head);
return 0;
}
```
阅读全文
相关推荐
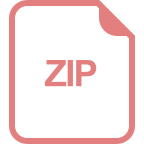



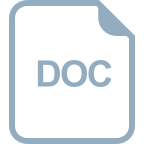
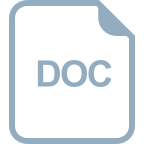
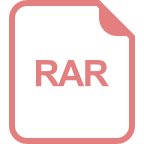
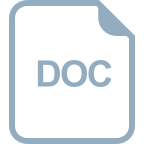








