vue3+typescript将token放在store中
时间: 2023-11-22 15:04:19 浏览: 158
在 Vue 3 + TypeScript 中,将 token 放在 Vuex store 中需要进行一些类型定义的操作。首先需要定义 `state` 中的类型和 `mutation` 的类型:
```typescript
// auth 模块
interface AuthState {
token: string | null;
}
interface AuthMutations {
setToken(state: AuthState, token: string): void;
}
const state: AuthState = {
token: null,
};
const mutations: AuthMutations = {
setToken(state, token) {
state.token = token;
},
};
```
接着,定义 `action` 的类型和参数类型:
```typescript
interface AuthActions {
login({ commit }: { commit: Commit }, { username, password }: { username: string, password: string }): Promise<void>;
logout({ commit }: { commit: Commit }): void;
}
const actions: AuthActions = {
async login({ commit }, { username, password }) {
// 发送登录请求并获取 token
const token = 'xxxxx';
commit('setToken', token);
},
logout({ commit }) {
// 清除 token
commit('setToken', null);
},
};
```
最后,定义 `store` 的类型和模块类型:
```typescript
import { createStore } from 'vuex';
interface RootState {}
interface Modules {
auth: {
state: AuthState;
mutations: AuthMutations;
actions: AuthActions;
};
}
export default createStore<RootState>({
modules: {
auth: {
state,
mutations,
actions,
},
},
});
```
这样,就可以在组件中使用类型安全的方式来访问和操作 token 了。例如,在组件中使用 `mapState` 辅助函数来将 token 映射到计算属性中:
```typescript
import { mapState } from 'vuex';
import { defineComponent } from 'vue';
export default defineComponent({
computed: {
...mapState('auth', ['token']),
},
});
```
在模板中,你可以直接使用 `{{ token }}` 来访问 token。在组件的方法中,也可以使用类型安全的方式来调用 `auth` 模块中的 action:
```typescript
import { mapActions } from 'vuex';
import { defineComponent } from 'vue';
export default defineComponent({
methods: {
...mapActions('auth', ['login']),
async handleLogin() {
await this.login({ username: 'xxx', password: 'xxx' });
},
},
});
```
这样,就可以在 Vue 3 + TypeScript 应用中安全地管理和使用 token 了。
阅读全文
相关推荐
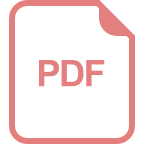
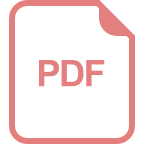



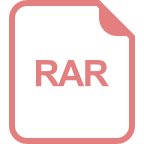
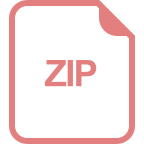
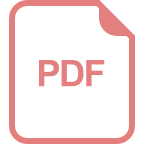
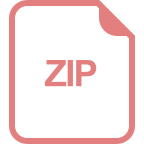
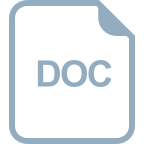
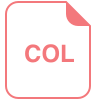
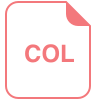





