用python 计算直接相邻的正负电荷基团之间的盐桥及其距离;一般也同时是氢键;
时间: 2024-02-23 12:59:39 浏览: 32
可以用Python中的生物信息学工具包Biopython来计算。具体步骤如下:
1. 读取蛋白质结构文件,比如PDB格式的文件。
```python
from Bio.PDB import PDBParser
parser = PDBParser()
structure = parser.get_structure('protein', 'protein.pdb')
```
2. 遍历结构中的原子,找到正负电荷基团(比如负电荷的羰基氧原子和正电荷的氨基氢原子),并计算它们之间的距离。
```python
from Bio.PDB.Polypeptide import is_aa, three_to_one
from Bio.PDB.NeighborSearch import NeighborSearch
# 用于判断原子是否为氨基氢或羰基氧
def is_charge(atom):
if atom.element == 'O' and atom.id == 'OXT':
return False
if atom.element == 'N' and atom.id == 'N' and atom.parent.resname == 'PRO':
return False
if atom.element == 'H' and atom.id == 'H':
return True
if atom.element == 'O' and atom.id == 'O':
return True
return False
# 遍历结构中的原子,找到正负电荷基团,并计算它们之间的距离
neighbor_search = NeighborSearch(list(structure.get_atoms()))
for residue in structure.get_residues():
if not is_aa(residue):
continue
resname = three_to_one(residue.resname)
for atom in residue.get_atoms():
if not is_charge(atom):
continue
neighbors = neighbor_search.search(atom.coord, 3.5) # 3.5埃为氢键的距离上限
for neighbor_atom in neighbors:
if not is_charge(neighbor_atom):
continue
if atom.parent == neighbor_atom.parent:
continue
neighbor_resname = three_to_one(neighbor_atom.parent.resname)
if resname == 'CYS' and neighbor_resname == 'CYS': # CYS之间形成二硫键
continue
distance = atom - neighbor_atom # 计算距离
print(f'{resname} {residue.id[1]} {atom.id} -> {neighbor_resname} {neighbor_atom.parent.id[1]} {neighbor_atom.id}: {distance:.2f} Å')
```
这个代码会输出所有形成盐桥或氢键的正负电荷基团之间的距离。
需要注意的是,这个代码中只考虑了直接相邻的正负电荷基团之间的相互作用,而实际上蛋白质结构中的盐桥和氢键可能会涉及到更远的原子。如果需要更全面地计算盐桥和氢键,可以使用更高级的生物信息学工具包,比如MDAnalysis。
相关推荐
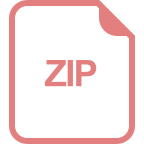
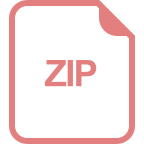














