用python实现卷积神经网络实现一个mnist手写数字识别
时间: 2023-06-10 09:04:46 浏览: 154
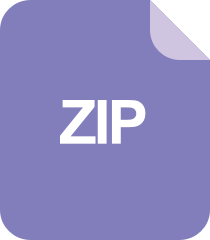
Python-使用卷积神经网络识别手写数字
好的,我可以帮你回答这个问题。要用Python实现卷积神经网络实现一个mnist手写数字识别,你可以按照以下步骤进行:
1.导入必要的库
使用Python中的import语句导入必要的库,例如tensorflow、keras、numpy等。
2.加载数据集
使用keras中的load_data()函数加载MNIST数据集。
3.预处理数据
对数据集进行预处理,例如将图像转换为灰度、归一化图像像素值等。
4.构建卷积神经网络模型
使用keras中的Sequential()函数创建卷积神经网络模型,在模型中添加卷积层、池化层、全连接层等。
5.编译模型
使用模型的compile()函数对模型进行编译,指定损失函数、优化器和评价指标等。
6.训练模型
使用模型的fit()函数对模型进行训练,指定训练数据和标签、批次大小、训练轮数等。
7.评估模型
使用模型的evaluate()函数对模型进行评估,指定测试数据和标签,输出模型的损失和精度等。
8.保存模型
使用keras中的save()函数保存训练好的模型。
希望这个回答对你有所帮助!
阅读全文
相关推荐
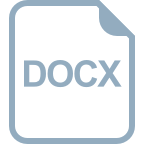


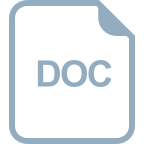
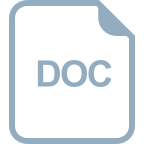

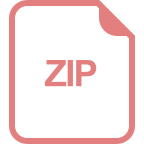
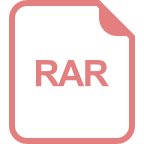
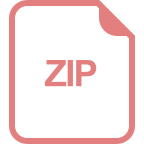
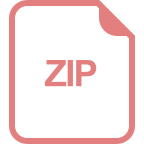
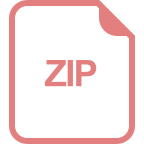




