改善一下上述对物资信息按采购人名字进行排序的代码
时间: 2024-03-03 12:50:25 浏览: 60
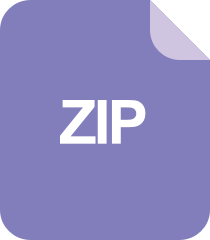
C语言程序设计大作业实现物资信息管理系统源代码.zip

当涉及到对结构体数组进行排序时,使用冒泡排序可能会很慢。以下是一个改进的排序算法,它使用快速排序(Quick Sort)算法来对结构体数组进行排序:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct {
char num[10];
char goods[10];
double price;
double count;
char cosumer[10];
} Material;
void swap(Material *a, Material *b) {
Material tmp = *a;
*a = *b;
*b = tmp;
}
int partition(Material arr[], int low, int high) {
char pivot[10];
strcpy(pivot, arr[high].cosumer);
int i = low - 1;
for (int j = low; j < high; j++) {
if (strcmp(arr[j].cosumer, pivot) < 0) {
i++;
swap(&arr[i], &arr[j]);
}
}
swap(&arr[i+1], &arr[high]);
return i + 1;
}
void quickSort(Material arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi-1);
quickSort(arr, pi+1, high);
}
}
int main() {
Material s[7] = {{"001", "goods1", 10.0, 20.0, "Cindy"},
{"002", "goods2", 20.0, 30.0, "Tom"},
{"003", "goods3", 30.0, 40.0, "Mary"},
{"004", "goods4", 40.0, 20.0, "Cindy"},
{"005", "goods5", 50.0, 30.0, "Tom"},
{"006", "goods6", 60.0, 40.0, "Mary"},
{"007", "goods7", 70.0, 20.0, "Cindy"}};
// 对结构体数组进行排序
quickSort(s, 0, 6);
// 输出排序后的结果
for (int i = 0; i < 7; i++) {
printf("%s %s %.2lf %.2lf %s\n", s[i].num, s[i].goods, s[i].price, s[i].count, s[i].cosumer);
}
return 0;
}
```
这个程序使用了快速排序算法来对结构体数组进行排序,时间复杂度为 O(nlogn),比冒泡排序的时间复杂度 O(n^2) 要快得多。
阅读全文
相关推荐
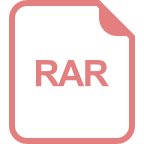
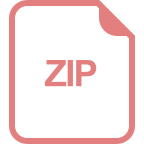
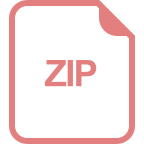
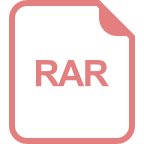
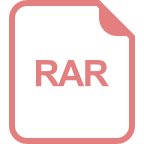
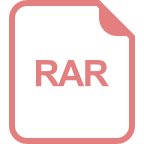
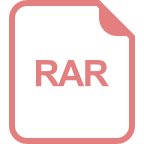
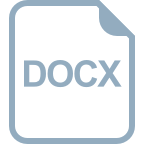
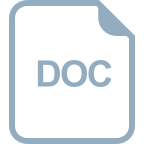
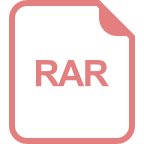
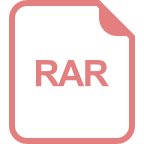
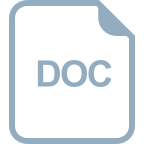
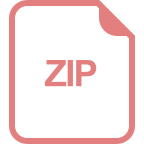