用c语言统计单链表L(带头结点)出现元素x的次数。
时间: 2023-12-20 22:03:40 浏览: 39
以下是统计单链表中某个元素出现次数的 C 语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义单链表结构体
typedef struct node {
int data;
struct node* next;
} Node, *LinkList;
// 统计元素 x 在链表 L 中出现的次数
int count(LinkList L, int x) {
int cnt = 0;
Node* p = L->next;
while (p != NULL) {
if (p->data == x) {
cnt++;
}
p = p->next;
}
return cnt;
}
int main() {
// 创建带头结点的单链表 L
LinkList L = (LinkList)malloc(sizeof(Node));
L->next = NULL;
Node* tail = L;
for (int i = 0; i < 10; i++) {
Node* p = (Node*)malloc(sizeof(Node));
p->data = i % 3; // 测试数据
p->next = NULL;
tail->next = p;
tail = p;
}
// 统计元素 x 在链表 L 中出现的次数
int x = 1; // 测试数据
int cnt = count(L, x);
printf("元素 %d 在链表 L 中出现了 %d 次。\n", x, cnt);
// 释放链表所占用的空间
Node* p = L->next;
while (p != NULL) {
Node* q = p;
p = p->next;
free(q);
}
free(L);
return 0;
}
```
代码思路是遍历单链表中的每个节点,如果节点的值等于 x,则计数器加一。最后返回计数器的值即为 x 在链表中出现的次数。
相关推荐
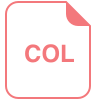
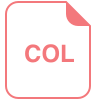
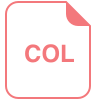
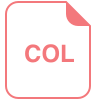
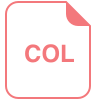









