c语言单链表程序代码带头结点,有头插入法和尾插入法的代码
时间: 2024-10-15 11:15:24 浏览: 35
在 C 语言中,单链表是一种动态数据结构,每个节点包含一个数据元素和指向下一个节点的指针。以下是创建单链表并实现头插法(insert at head)和尾插法(insert at tail)的基本示例,这里假设有一个结构体 Node 和一个函数声明:
```c
// 定义链表节点结构体
typedef struct Node {
int data; // 节点存储的数据
struct Node* next; // 指向下一个节点的指针
} Node;
// 初始化头节点
Node* createList() {
Node* head = (Node*)malloc(sizeof(Node));
if (head == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
head->data = 0; // 额外处理头节点,通常设为特殊值或NULL
head->next = NULL; // 初始化头节点的 next 指针
return head;
}
// 头部插入节点
void insertAtHead(Node** head_ref, int value) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 创建新节点
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
newNode->next = *head_ref; // 新节点的 next 指向当前头节点
*head_ref = newNode; // 更新头节点指针
}
// 尾部插入节点(双指针法)
void insertAtTail(Node** head_ref, int value) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 创建新节点
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
// 如果链表为空,则直接将头和尾设置为新节点
if (*head_ref == NULL) {
*head_ref = newNode;
return;
}
Node* temp = *head_ref; // 临时指针,用于遍历到链表尾部
while (temp->next != NULL) {
temp = temp->next; // 跟随现有节点到链尾
}
temp->next = newNode; // 将新节点添加到链表尾部
}
// 打印链表
void printList(Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
int main() {
Node* head = createList();
insertAtHead(&head, 5);
insertAtTail(&head, 10);
printList(head);
return 0;
}
```
阅读全文
相关推荐
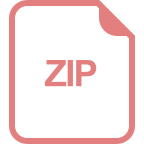
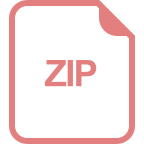
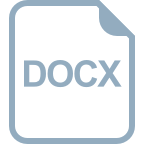
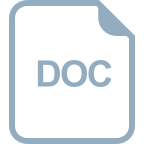



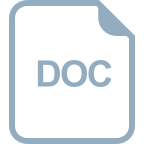
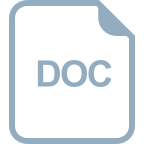









