c++ float 字符串
时间: 2023-10-05 07:09:58 浏览: 98
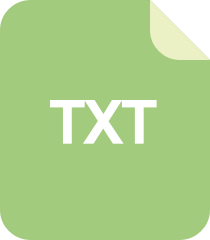
c++字符串转换大全

你可以使用 `std::stof` 函数将字符串转换为浮点数。示例代码如下:
```c++
#include <iostream>
#include <string>
int main() {
std::string str = "3.14";
float f = std::stof(str);
std::cout << f << std::endl; // 输出 3.14
return 0;
}
```
请注意,在转换字符串时,`std::stof` 函数会尝试将字符串转换为浮点数。如果字符串无法转换为浮点数,则会抛出 `std::invalid_argument` 异常。如果浮点数超出了可表示的范围,则会抛出 `std::out_of_range` 异常。因此在实际使用时,需要进行异常处理。
阅读全文
相关推荐
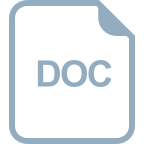
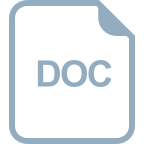

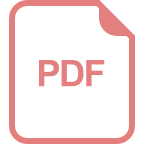
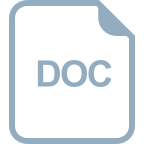












