qt实现哨兵模式具体代码
时间: 2023-11-15 20:49:10 浏览: 219
哨兵模式(Sentinel Pattern)是一种软件设计模式,用于在软件系统中处理边界条件。在Qt中实现哨兵模式可以使用QAbstractItemModel类的rowCount()和columnCount()方法来判断边界条件。
以下是一个简单的示例代码,用于演示如何在Qt中实现哨兵模式:
```c++
#include <QAbstractItemModel>
#include <QModelIndex>
class MyModel : public QAbstractItemModel
{
public:
MyModel(QObject *parent = nullptr) : QAbstractItemModel(parent) {}
// 实现rowCount()方法
int rowCount(const QModelIndex &parent = QModelIndex()) const override
{
if (parent.isValid()) {
return 0; // 使用哨兵模式,返回0
} else {
return m_rows; // 返回实际的行数
}
}
// 实现columnCount()方法
int columnCount(const QModelIndex &parent = QModelIndex()) const override
{
if (parent.isValid()) {
return 0; // 使用哨兵模式,返回0
} else {
return m_columns; // 返回实际的列数
}
}
// 实现index()方法
QModelIndex index(int row, int column, const QModelIndex &parent = QModelIndex()) const override
{
if (row < 0 || row >= m_rows || column < 0 || column >= m_columns) {
return QModelIndex(); // 使用哨兵模式,返回空的QModelIndex
} else {
return createIndex(row, column); // 返回一个有效的QModelIndex
}
}
// 实现parent()方法
QModelIndex parent(const QModelIndex &child) const override
{
Q_UNUSED(child)
return QModelIndex(); // 使用哨兵模式,返回空的QModelIndex
}
// 实现data()方法
QVariant data(const QModelIndex &index, int role = Qt::DisplayRole) const override
{
if (!index.isValid()) {
return QVariant();
}
if (role != Qt::DisplayRole) {
return QVariant();
}
return QString("row %1, column %2").arg(index.row()).arg(index.column());
}
private:
int m_rows = 10;
int m_columns = 5;
};
```
在上面的示例代码中,我们使用了哨兵模式来处理rowCount()、columnCount()、index()和parent()方法中的边界条件。当传递给这些方法的QModelIndex对象是无效的时候,我们返回了一个空的QModelIndex对象或者0,以表示哨兵模式。在data()方法中,我们只返回了DisplayRole角色的数据,用于在QTableView中显示数据。
阅读全文
相关推荐
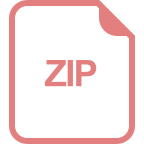
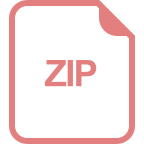
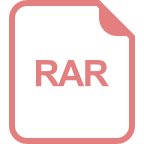
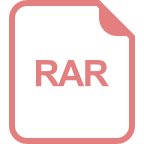
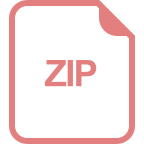
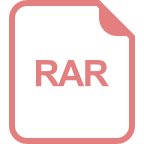
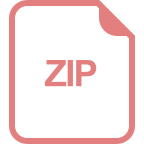
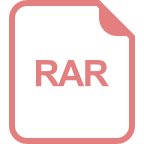
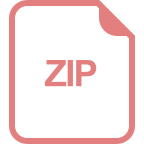
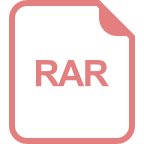
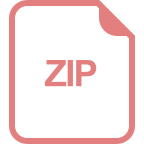
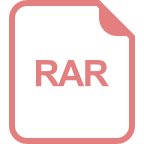
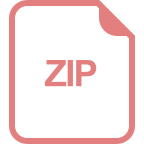
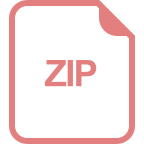
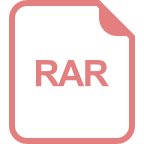
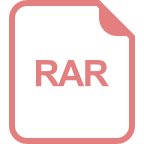