从虚基类 Plane 派生出具体类(如长方形类 Rectangle、圆形类 Circle 和三角形类tr
时间: 2024-05-02 19:16:59 浏览: 137
iangle),并在每个类中添加特定的成员函数和成员变量,以实现对该类图形的基本操作。同时,为了保证类的一致性和可扩展性,还可以在 Plane 类中定义虚函数,使得派生类可以根据自身的特点来实现该函数。例如:
```c++
class Plane {
public:
virtual double area() const = 0; // 纯虚函数,用于计算图形面积
virtual double perimeter() const = 0; // 纯虚函数,用于计算图形周长
virtual void draw() const = 0; // 纯虚函数,用于绘制图形
};
class Rectangle : public Plane {
public:
Rectangle(double w, double h) : width(w), height(h) {}
double area() const override { return width * height; }
double perimeter() const override { return 2 * (width + height); }
void draw() const override { /* 绘制长方形 */ }
private:
double width;
double height;
};
class Circle : public Plane {
public:
Circle(double r) : radius(r) {}
double area() const override { return 3.14159 * radius * radius; }
double perimeter() const override { return 2 * 3.14159 * radius; }
void draw() const override { /* 绘制圆形 */ }
private:
double radius;
};
class Triangle : public Plane {
public:
Triangle(double a, double b, double c) : sideA(a), sideB(b), sideC(c) {}
double area() const override {
double p = (sideA + sideB + sideC) / 2;
return sqrt(p * (p - sideA) * (p - sideB) * (p - sideC));
}
double perimeter() const override { return sideA + sideB + sideC; }
void draw() const override { /* 绘制三角形 */ }
private:
double sideA, sideB, sideC;
};
```
这样,我们就可以通过多态的方式,使用基类指针或引用来处理不同的图形对象,而无需关注具体对象的类型。例如:
```c++
Plane* shape = new Rectangle(2.0, 3.0);
std::cout << "Rectangle area: " << shape->area() << std::endl; // 输出 6.0
shape = new Circle(4.0);
std::cout << "Circle perimeter: " << shape->perimeter() << std::endl; // 输出 25.1327
```
通过这种方式,我们可以将不同的图形对象统一地处理,提高了代码的可复用性和可扩展性。
阅读全文
相关推荐





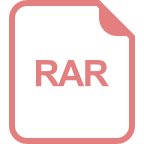











