以上程序不使用stdib.h
时间: 2024-03-01 14:54:41 浏览: 25
好的,以下是不依赖于 `stdlib.h` 的实现:
```
#include <stdio.h>
#include <string.h>
#define MAX_LEN 20
#define MAX_NUM 10
// 定义日期结构体
typedef struct {
int year;
int month;
int day;
} Date;
// 定义联系人结构体
typedef struct {
char name[MAX_LEN];
Date birthday;
char phone[MAX_LEN];
} Person;
// 比较函数,按年龄从大到小排序
int cmp(Person a, Person b) {
if (a.birthday.year != b.birthday.year) {
return b.birthday.year - a.birthday.year;
} else if (a.birthday.month != b.birthday.month) {
return b.birthday.month - a.birthday.month;
} else {
return b.birthday.day - a.birthday.day;
}
}
// 冒泡排序
void bubble_sort(Person people[], int n) {
for (int i = 0; i < n; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (cmp(people[j], people[j + 1]) > 0) {
Person temp = people[j];
people[j] = people[j + 1];
people[j + 1] = temp;
}
}
}
}
int main() {
int n;
Person people[MAX_NUM];
printf("请输入联系人数量(不超过10个):");
scanf("%d", &n);
if (n <= 0 || n > MAX_NUM) {
printf("输入错误!\n");
return 0;
}
printf("请分别输入联系人的姓名、生日(年 月 日)、电话号码:\n");
for (int i = 0; i < n; i++) {
scanf("%s %d %d %d %s", people[i].name, &people[i].birthday.year, &people[i].birthday.month, &people[i].birthday.day, people[i].phone);
}
// 排序
bubble_sort(people, n);
// 输出
for (int i = 0; i < n; i++) {
printf("%s %04d%02d%02d %s\n", people[i].name, people[i].birthday.year, people[i].birthday.month, people[i].birthday.day, people[i].phone);
}
return 0;
}
```
这里使用了冒泡排序进行排序。冒泡排序是比较简单的一种排序算法,虽然时间复杂度较高,但对于数据规模较小的情况,其性能表现不错。
相关推荐
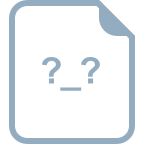
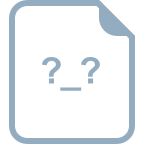
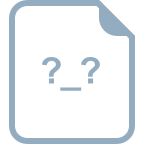
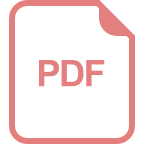
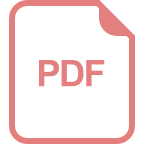
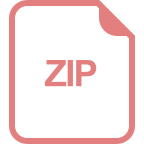
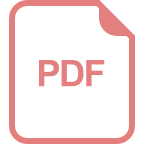
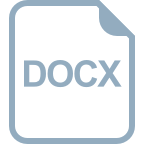
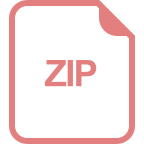
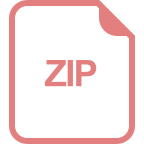
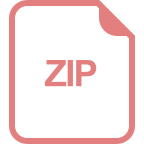
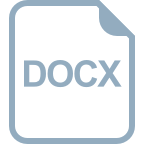
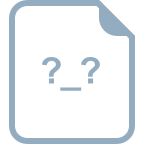