帮我写一个用c++下用opencv4.6 dlib19 基于facenet实现的人脸比对项目
时间: 2023-11-27 14:54:51 浏览: 97
好的,以下是一个简单的人脸比对项目,需要注意的是,这个项目只是一个示例,你需要根据自己的实际需求进行修改和完善。
首先,你需要安装并配置好 OpenCV 和 dlib 库,这里不再赘述。
然后,你需要下载 facenet 的预训练模型文件,可以在官网上找到下载链接。
接下来是代码实现:
```cpp
#include <iostream>
#include <opencv2/opencv.hpp>
#include <dlib/dnn.h>
#include <dlib/opencv.h>
using namespace std;
using namespace cv;
using namespace dlib;
using namespace dlib::dnn;
int main(int argc, char** argv) {
// 加载 facenet 模型
net_type net;
deserialize("models/facenet_pretrained.dat") >> net;
// 加载图片并进行人脸检测和对齐
frontal_face_detector detector = get_frontal_face_detector();
shape_predictor sp;
deserialize("models/shape_predictor_5_face_landmarks.dat") >> sp;
Mat img1 = imread("img1.jpg", IMREAD_GRAYSCALE);
Mat img2 = imread("img2.jpg", IMREAD_GRAYSCALE);
std::vector<dlib::rectangle> dets1 = detector(to_image_gray(img1));
std::vector<dlib::rectangle> dets2 = detector(to_image_gray(img2));
std::vector<full_object_detection> shapes1;
std::vector<full_object_detection> shapes2;
for (unsigned long j = 0; j < dets1.size(); ++j) {
full_object_detection shape = sp(to_image_gray(img1), dets1[j]);
shapes1.push_back(shape);
}
for (unsigned long j = 0; j < dets2.size(); ++j) {
full_object_detection shape = sp(to_image_gray(img2), dets2[j]);
shapes2.push_back(shape);
}
// 提取人脸特征并计算相似度
matrix<rgb_pixel> img1_aligned, img2_aligned;
extract_image_chip(to_image(img1), get_face_chip_details(shapes1[0], 150, 0.25), img1_aligned);
extract_image_chip(to_image(img2), get_face_chip_details(shapes2[0], 150, 0.25), img2_aligned);
matrix<rgb_pixel> img1_rgb, img2_rgb;
assign_image(img1_rgb, img1_aligned);
assign_image(img2_rgb, img2_aligned);
matrix<float, 0, 1> face1 = net(img1_rgb);
matrix<float, 0, 1> face2 = net(img2_rgb);
float score = dot(face1, face2) / (length(face1) * length(face2));
std::cout << "Similarity score: " << score << std::endl;
return 0;
}
```
这段代码的功能是比较两张图片中人脸的相似度,具体实现如下:
1. 加载 facenet 模型,用于提取人脸特征。
2. 使用 dlib 库的人脸检测器和关键点检测器,对输入的图片进行人脸检测和对齐。
3. 提取人脸图像并转换为 facenet 所需的 RGB 矩阵格式。
4. 使用模型提取人脸特征,并计算相似度。
需要注意的是,这段代码只比较了两张图片中的第一张人脸,如果需要比较多张图片中的多个人脸,需要对代码进行相应的修改。
另外,这段代码只是一个示例,实际应用中可能需要对人脸图像进行更多的预处理,例如去除噪声、对比度增强等等,以提高识别的准确率。
阅读全文
相关推荐
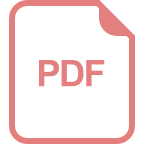
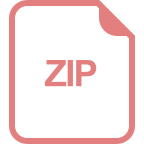
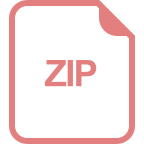
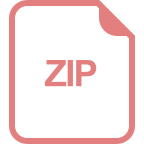
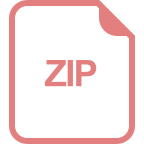
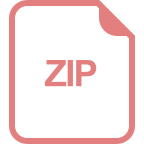
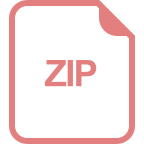
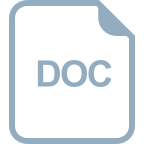
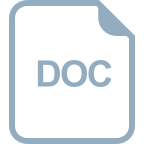







