用Python写一个保留五天的以时间命名的文件夹内的PNG格式图片和保留30天的JPG格式的图片的脚本
时间: 2023-08-31 08:34:46 浏览: 72
### 回答1:
可以这么做:import os
import time# 获取当前目录下的所有文件
files = os.listdir('.')# 遍历文件
for file in files:
# 获取文件的创建时间
time_created = os.path.getctime(file)
# 计算文件创建时距离当前时间的天数
day_passed = (time.time() - time_created) // 86400
# 如果文件是PNG格式的
if file.endswith('.png'):
# 如果创建时间距离当前时间超过五天,则删除文件
if day_passed > 5:
os.remove(file)
# 如果文件是JPG格式的
elif file.endswith('.jpg'):
# 如果创建时间距离当前时间超过30天,则删除文件
if day_passed > 30:
os.remove(file)
### 回答2:
以下是使用Python编写的脚本,可实现保留五天的以时间命名的文件夹内的.png格式图片和保留三十天的.jpg格式图片的功能。
```python
import os
import time
def delete_old_files(folder, days_to_keep, file_extension):
current_time = time.time()
for file in os.listdir(folder):
file_path = os.path.join(folder, file)
if file.endswith("." + file_extension):
creation_time = os.path.getctime(file_path)
time_difference = current_time - creation_time
days_difference = time_difference / (60 * 60 * 24)
if days_difference > days_to_keep:
os.remove(file_path)
def organize_files(source_folder, destination_folder):
current_time = time.strftime("%Y-%m-%d", time.localtime())
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
for file in os.listdir(source_folder):
file_path = os.path.join(source_folder, file)
if file.endswith(".png"):
new_folder = os.path.join(destination_folder, "png")
elif file.endswith(".jpg"):
new_folder = os.path.join(destination_folder, "jpg")
else:
continue # Ignore other file types
if not os.path.exists(new_folder):
os.makedirs(new_folder)
new_file_name = os.path.join(new_folder, current_time + "_" + file)
os.rename(file_path, new_file_name)
if __name__ == "__main__":
source_folder = "path/to/source/folder"
destination_folder = "path/to/destination/folder"
delete_old_files(destination_folder, 5, "png")
delete_old_files(destination_folder, 30, "jpg")
organize_files(source_folder, destination_folder)
```
以上脚本首先定义了两个功能函数:
1. `delete_old_files`:用于删除超过指定天数的文件。
2. `organize_files`:用于将源文件夹中的文件按照格式命名并移动到目标文件夹中。
在主函数中,我们指定源文件夹`source_folder`和目标文件夹`destination_folder`。然后分别使用`delete_old_files`函数删除目标文件夹中超过五天的".png"格式文件和超过三十天的".jpg"格式文件。最后,使用`organize_files`函数将源文件夹中的文件按照格式命名并移动到目标文件夹中。
请根据实际需求修改源文件夹和目标文件夹的路径,并确保已安装Python环境并将脚本保存为.py文件,在命令行中运行该文件即可实现相应功能。
### 回答3:
可以使用Python中的os、datetime和shutil模块来编写一个脚本来实现这个需求。
首先,我们需要导入所需的模块:
```python
import os
import datetime
import shutil
```
然后,我们可以定义目标文件夹的路径和相应的日期范围:
```python
folder_path = "/path/to/folder"
# 保留五天内的PNG文件夹
png_folder = datetime.datetime.now().strftime("%Y-%m-%d")
png_expiration_date = (datetime.datetime.now() - datetime.timedelta(days=5)).strftime("%Y-%m-%d")
# 保留30天内的JPG文件夹
jpg_folder = datetime.datetime.now().strftime("%Y-%m-%d")
jpg_expiration_date = (datetime.datetime.now() - datetime.timedelta(days=30)).strftime("%Y-%m-%d")
```
接下来,我们可以遍历目标文件夹中的所有文件,然后根据文件的类型和日期将其移动到相应的子文件夹:
```python
for file in os.listdir(folder_path):
if file.endswith(".png"):
file_path = os.path.join(folder_path, file)
# 如果文件的创建日期在五天内,将其移动到PNG文件夹
if datetime.datetime.fromtimestamp(os.stat(file_path).st_birthtime).strftime("%Y-%m-%d") >= png_expiration_date:
destination_path = os.path.join(folder_path, png_folder)
os.makedirs(destination_path, exist_ok=True)
shutil.move(file_path, destination_path)
elif file.endswith(".jpg"):
file_path = os.path.join(folder_path, file)
# 如果文件的创建日期在三十天内,将其移动到JPG文件夹
if datetime.datetime.fromtimestamp(os.stat(file_path).st_birthtime).strftime("%Y-%m-%d") >= jpg_expiration_date:
destination_path = os.path.join(folder_path, jpg_folder)
os.makedirs(destination_path, exist_ok=True)
shutil.move(file_path, destination_path)
```
最后,脚本会将在五天内创建的PNG文件移动到以当前日期命名的PNG文件夹,以及将在30天内创建的JPG文件移动到以当前日期命名的JPG文件夹。
注意:该脚本假设文件的创建日期可以通过os.stat().st_birthtime获取,并且文件的命名方式为YYYY-MM-DD.png和YYYY-MM-DD.jpg。如果您的系统不支持获取创建日期或者文件命名方式不同,请相应地进行修改。
相关推荐
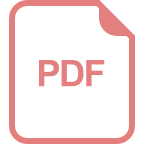
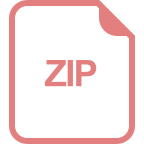
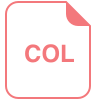
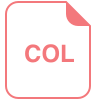
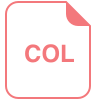
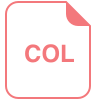
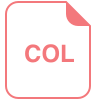









