vue3在setup中取props的值
时间: 2023-05-13 22:04:39 浏览: 79
可以使用ES6的解构赋值来取props的值,例如:
```
setup(props) {
const { prop1, prop2 } = toRefs(props);
// prop1和prop2就是父组件传递过来的props值
}
```
相关问题
如何在vue3 setup 中使用props向子组件传递数据
你可以在父组件的template中使用子组件并给它们添加需要传递的属性,然后在子组件中使用props来读取这些属性的值。在vue3 setup中,你可以通过使用ref函数来定义props和对其进行赋值,然后在模板中使用组件并将props属性传递给它们。例如:
在父组件中:
```
<template>
<child-component :message="msg" />
</template>
<script>
import { ref } from 'vue'
import ChildComponent from './ChildComponent.vue'
export default {
components: {
ChildComponent
},
setup() {
const msg = ref('Hello, child component!')
return {
msg
}
}
}
</script>
```
在子组件中:
```
<template>
<div>{{ message }}</div>
</template>
<script>
import { defineProps } from 'vue'
export default {
props: {
message: {
type: String,
required: true
}
},
setup(props) {
defineProps({
message: props.message
})
}
}
</script>
```
这样,在父组件中的msg变量的值就可以通过props的形式传递到子组件中,并在子组件中使用。
vue3 setup 如何获取 props
在 Vue 3 中,使用 `setup()` 钩子编写组件时,你可以直接从 `this` 上访问 props。`this` 关联到当前组件实例,所以你可以像这样获取 props:
```vue
<template>
<div>
<!-- 在模板中使用 props -->
{{ message }}
<!-- 可能还有其他元素,如按钮,根据 prop 更新内容 -->
</div>
</template>
<script setup>
import { ref, computed } from 'vue'; // 引入必要的依赖
// 假设我们有一个名为 "message" 的 prop
const message = this.$props.message; // 直接从 this 访问 prop
// 或者使用 computed 函数包装,确保只在需要时更新
const formattedMessage = computed(() => {
return `你好,${message}`;
});
</script>
```
在上面的例子中,`$props` 对象包含了所有的传给组件的 props。注意,在 `setup` 中,你需要导入 `ref` 和 `computed` 或其他相关的库来处理可能的计算属性。
阅读全文
相关推荐
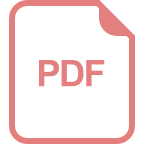
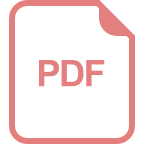
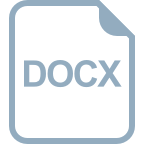
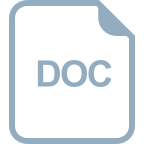










