写一段图片上传的C++代码 并且实现HTTP POST请求
时间: 2023-03-03 09:13:10 浏览: 191
以下是一个使用C语言编写的基本图片上传代码,实现HTTP POST请求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <curl/curl.h>
int main(int argc, char *argv[]) {
CURL *curl;
CURLcode res;
FILE *file;
char *url = "http://example.com/upload_image.php"; // 上传图片的URL地址
char *filename = "example.jpg"; // 需要上传的图片文件名
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, url);
curl_easy_setopt(curl, CURLOPT_POST, 1L);
// 设置上传文件的参数
struct curl_httppost *formpost = NULL;
struct curl_httppost *lastptr = NULL;
curl_formadd(&formpost, &lastptr,
CURLFORM_COPYNAME, "image",
CURLFORM_FILE, filename,
CURLFORM_END);
curl_easy_setopt(curl, CURLOPT_HTTPPOST, formpost);
res = curl_easy_perform(curl);
if(res != CURLE_OK) {
fprintf(stderr, "curl_easy_perform() failed: %s\n",
curl_easy_strerror(res));
}
// 清理上传文件的参数
curl_formfree(formpost);
curl_easy_cleanup(curl);
}
return 0;
}
```
以上代码使用了libcurl库,可以使用`curl_easy_setopt`函数设置HTTP请求的参数,使用`curl_formadd`函数设置上传文件的参数。具体来说,使用`CURLFORM_COPYNAME`指定上传文件的参数名,使用`CURLFORM_FILE`指定需要上传的文件名。最后使用`curl_easy_perform`函数执行HTTP请求,并使用`curl_formfree`函数清理上传文件的参数。
需要注意的是,以上代码仅供参考,具体实现需要根据具体的情况进行修改。特别是需要根据实际情况设置上传图片的URL地址和需要上传的图片文件名。
相关推荐
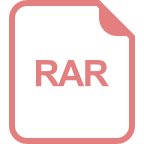
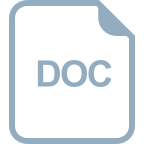
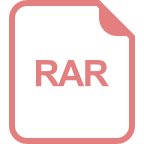














